Root mean square of a function in python
115,268
Solution 1
I'm going to assume that you want to compute the expression given by the following pseudocode:
ms = 0
for i = 1 ... N
ms = ms + y[i]^2
ms = ms / N
rms = sqrt(ms)
i.e. the square root of the mean of the squared values of elements of y
.
In numpy, you can simply square y
, take its mean and then its square root as follows:
rms = np.sqrt(np.mean(y**2))
So, for example:
>>> y = np.array([0, 0, 1, 1, 0, 1, 0, 1, 1, 1]) # Six 1's
>>> y.size
10
>>> np.mean(y**2)
0.59999999999999998
>>> np.sqrt(np.mean(y**2))
0.7745966692414834
Do clarify your question if you mean to ask something else.
Solution 2
You could use the sklearn function
from sklearn.metrics import mean_squared_error
rmse = mean_squared_error(y_actual,[0 for _ in y_actual], squared=False)
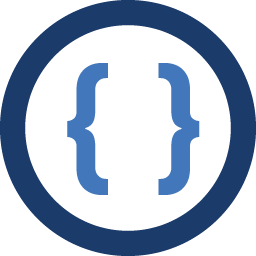
Author by
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I want to calculate root mean square of a function in Python. My function is in a simple form like y = f(x). x and y are arrays.
I tried Numpy and Scipy Docs and couldn't find anything.
-
Praveen over 7 yearsWhat is the exact function you are trying to compute? Root-mean-squared value of y?
-
Praveen over 7 yearsIt would also help if you could add an example array and expected solution...
-
-
Admin over 7 yearsThank you very much. I still wonder something. The definition part in wikipedia says, the RMS is actually a limit. I mean it goes on and on. But in your answer, only first degree was regarded. I think we neglect the rest somehow during calculation? Your answer works. But I ask this to clarify the whole subject.
-
Praveen over 7 years@ordinary If you are trying to calculate the RMS value of a sine wave (i.e. f(x) is a sine function) or some such, then you need to perform an integration. This is approximated as a summation here. The limit comes in because you need to take infinitesimally small intervals for your sum. In other words, you can get a better estimate of the RMS value if you use a finer resolution in
x
. -
PatriceG almost 7 yearsWarning: in numpy, if the number are too big in comparison of their type (dtype in python), the power function may return negative values. To avoid this, it is sometimes useful to cast the values. Example: >>np.sqrt(np.mean(y.astype(np.dtype(np.int64)) ** 2 )). Not very nice in the code, but it will do the work!
-
Praveen almost 7 years@sandoval31 You probably want to type-cast into float though, not into a bigger integer. Since RMS is in general a non-integer anyway, you're better off doing
y.astype(float)
.