Routes.push() not working as expected at NextJS
Solution 1
It seems you were using next-router dependecy?
Try to use next/router
(default dependency from nextjs), it works fine in my code:
import Router from "next/router";
{ other code... }
Router.push("/auth/signup");
Solution 2
I have the same problem and found a workaround, but not a solution. I use window.location
//keep your base urls in a global variables file
import { devUri, productionUri } from "../globalVariables";
push = (route = "") => {
const uri = process.env.NODE_ENV === "production" ? productionUri : devUri;
window.location = uri + '/' + route
}
push("/auth/signup")
push("/path/to/whatever/route/you/like")
push() //navigates to your base uri
}
You could save that function into your version of globalVariables.js
, and import as needed (much the same way you import other functions from 3rd party modules)
import { devUri, productionUri, push } from "../globalVariables";
BTW, I run into the exact same issues you're having: Router.push("/") or any other address that exists just doesn't seem to fire at all, Router.push("/unexistentlocation") works normally
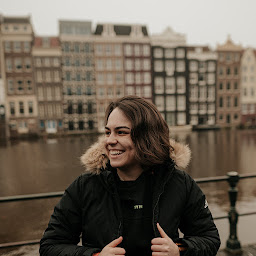
Comments
-
Laura Beatris 7 months
I am trying to redirect the user to the homepage when he tries to access the dashboard of the app without being authenticated, but the Route.push() is not working.
I tried to change Router.push('/') to Router.push('/hey') -> a page that does not exists, and this worked, the route changed. Also when I try to access a path like this Router.push('/auth/signin'), it doesn't work while Router.push('/auth') works
import { isAuthenticated } from "../helpers/auth"; import { Component } from "react"; import { Router } from "../routes"; class Ads extends Component { // static async getInitialProps(ctx) { // if (ctx && ctx.req) { // console.log(ctx); // //ctx.res.writeHead(302, { Location: `/` }); // ctx.res.end(); // } else { // console.log("client side"); // console.log(localStorage.getItem("auth-token")); // } // } handleAuthentication = () => { if (localStorage.getItem("auth-token")) { return true; } }; componentDidMount() { if (this.handleAuthentication()) { console.log("hey"); } else { Router.pushRoute("/auth/signup"); } } render() { return ( <div> <p> Dashboard </p> </div> ); } } export default Ads;
-
Laura Beatris over 3 yearsNow i changed to Router.push("/ads", "/"), and the url is changing but is not redirecting. That's my repo: github.com/LauraBeatris/befree-front
-
Darryl RN over 3 yearsHave you tried to add the third parameter shallow true? Seems you want to use an alias, read the documentation here github.com/zeit/next.js#shallow-routing
Router.push(href, as, { shallow: true })
-
Laura Beatris over 3 yearsNow i did this: ` componentDidMount() { const as = "/"; if (!localStorage.getItem("auth-token")) { Router.push("/ads", as, { shallow: true }); //window.location.reload(); } } ` And still just changing the url, like, localhost:3000/ads to localhost:3000, thats what i want, but the page still on the same place
-
Mario over 2 yearsI am experimenting the same problem, Any idea how to fix it?
-
Darryl RN over 2 years@LauraBeatris I'm able to use
next/router
and redirect to yourauth/signin
in your repo. I replaceimport { Router } from "../routes";
withimport Router from "next/router";
. Then addRouter.push("/auth/signin");
in ln.28 and it works. Redirect unauthorized user to your signin page. -
Darryl RN over 2 years@user615274 what is your problem? using the same
next-router
dependency? -
Wizard 6 monthsCheck your console, you may have a runtime error preventing the page from proceeding. In my case I had an error "Maximum update depth exceeded" which was stopping router.push from completing