Ruby on Rails: wrong number of arguments (given 0, expected 1)
12,699
You need to pass produit_id as a parameter.
So change this line as so...
<%= link_to 'Ajouter au panier',
carts_add_item_path(produit_id: produit.id), method: :post %>
And change your controller method as so...
def add_item
produit_id = params[:produit_id]
...
And change the find_or_create to
@cart_item = CartItem.find_or_create_by(produit_id: produit_id)
This does mean that you can only have one CartItem in your entire application that points to a product... strange design.
Related videos on Youtube
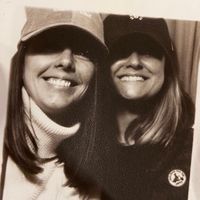
Author by
justinedps26
Updated on June 04, 2022Comments
-
justinedps26 7 months
I have an issue with my
add_item
method and have trouble to understand why.Here is my
carts_controller.rb
class CartsController < ApplicationController def index @cart_items = CartItem.all end def add_item @cart_item = CartItem.new produit_id = params[:produit_id] @cart_item = CartItem.find_or_create_by(params[:produit][:produit_id]) @cart_item.save binding.pry end end
Here is
produits/index.html.erb
(where the issue comes from)<div id="produits-column-container"> <% if @produits %> <% @produits.in_groups_of(4, false).each do |g| %> <% g.each do |produit| %> <div id="produits-row-container"> <div id="fiche-produit-container"> <div id="produit-img"> <%= image_tag produit.photo %> </div> <div id="produit-nom"> <%= produit.nom %> </div> <div id="produit-prix"> <%= number_to_currency(produit.prix, unit: '€', format: "%n%u") %> </div> <div id="produit-au-panier"> <%= image_tag('icon/icon-panier') %> <%= link_to 'Ajouter au panier', carts_add_item_path, method: :post %> </div> </div> </div> <% end %> <% end %> <% end %> </div>
The error i'm given is :
ArgumentError in CartsController#add_item wrong number of arguments (given 0, expected 1) in def add_item(produit_id)
add_item(produit_id)
is related tocarts_add_item_path
I also give you the routes :
Rails.application.routes.draw do match "/mon-panier" => 'carts#index', via: :get post 'carts/add_item' => 'carts#add_item' resources :categories do resources :produits end resources :order_abonnements, only: [:create, :update, :delete] get 'livraisons_type/index' match "/recapitulatif" => 'recapitulatif#index', via: :get match "/confirmation-carte-cadeau" => 'recapitulatif#confirmation', via: :get match "/livraison-carte-cadeau" => 'livraison_carte#index', via: :get match '/activation-carte' => 'code_carte_cadeau#index', via: :get match "/offrir-une-box-bretonne" => 'cadeau#index', via: :get resources :order_items, only: [:create, :update, :destroy] match "/nos-box" => 'nos_box#index', via: :get get 'categories/index' devise_for :admin_users, ActiveAdmin::Devise.config ActiveAdmin.routes(self) match '/informations-penn-ar-box' => 'informations_penn_ar_box#index', via: :get match '/livraison-box-bretonne' => 'livraison_box_bretonne#index', via: :get match '/abonnements' => 'abonnements#index', via: :get devise_for :users, path: '', path_names: { sign_in: 'connexion', sign_out: 'déconnexion'} resources :users do delete 'déconnexion' => 'devise/sessions#destroy' end match '/mon-marche-breton' => 'marche_breton#index', via: :get root 'home#home' end
And the logs :
Started POST "/carts/add_item" for ::1 at 2017-05-30 09:48:52 +0200 Processing by CartsController#add_item as HTML Parameters: {"authenticity_token"=>"QrToQUHVxjuV5cUvZYHd7tj457htfZohOkmsvNDnKv79P413xjsSfR/8RVXtdIU7/wcmhcxjkU85N13CqJkG2w=="} Cart Load (0.3ms) SELECT `carts`.* FROM `carts` WHERE `carts`.`id` = 1 LIMIT 1 Completed 500 Internal Server Error in 27ms (ActiveRecord: 14.9ms) ArgumentError (wrong number of arguments (given 0, expected 1)): app/controllers/carts_controller.rb:6:in `add_item'
-
Sergio Tulentsev over 5 yearsController actions are not your ordinary ruby methods and they don't accept parameters like that, in the signature. You're supposed to read
params[:produit_id]
in the method body. -
Aleksei Matiushkin over 5 years@SergioTulentsev well, controller actions are ordinary ruby methods after all, they just are called by the engine in some ways that the engine wants to call them.
-
Sergio Tulentsev over 5 years@mudasobwa: you know what I meant. :)
-
justinedps26 over 5 years@SergioTulentsev what do i need to write to fix the issue? i'm in a hurry :)
-
Sergio Tulentsev over 5 years@justinedps26: the answer is in the last sentence of my comment
-
justinedps26 over 5 years@SergioTulentsev i did write your way but nothing changed
-
Sergio Tulentsev over 5 years@justinedps26 you forgot to remove the parameter from the signature?
-
Sergio Tulentsev over 5 years@justinedps26:
def add_item(produit_id)
should bedef add_item
-
zauzaj over 5 years@justinedps26 as @SergioTulentsev explained, controller actions don't receive parameters; as you can see you even don't use such parameter in action body but you access to
params
. So just remove parameter from method signature and bug will be solved ! -
justinedps26 over 5 years@SergioTulentsev now i have
undefined local variable or method
cart_items'` -
Sergio Tulentsev over 5 years@justinedps26: well, that's another issue, which doesn't have anything to do with the original error.
-
justinedps26 over 5 years@SergioTulentsev well, i'll try to figure it out. Thanks for your help
-
Sergio Tulentsev over 5 yearsNow the question is wrong. The code given can't produce stated error. And, as a general rule, you must not edit questions like that (so that it becomes another question entirely). This invalidates existing answers.
-
justinedps26 over 5 years@SergioTulentsev okay sorry, i just did that to be more clear in the comments
-
Sergio Tulentsev over 5 years@justinedps26: no problem. But do keep that in mind.
-
justinedps26 over 5 yearsLet us continue this discussion in chat.
-
-
Sergio Tulentsev over 5 yearsThat'll be useful, yes, but it's not the cause of the exact problem in the question.
-
justinedps26 over 5 yearsThanks, can you let me know the other things that are wrong please?
-
SteveTurczyn over 5 years? @SergioTulentsev surely it is? He's
calling carts_add_item_path
which resolves into a call toadd_item
without arguments. HIs definition of theadd_item
action expects an argument. Wrong number of arguments, given 0, expected 1. -
Sergio Tulentsev over 5 yearsNo, invocation of
carts_add_item_path
does not end up with a call toadd_item
. -
Sergio Tulentsev over 5 yearsAh, I see where I could be misunderstood. I was referring to the first part of the answer, the one with
carts_add_item_path(produit_id: produit.id)
. If you do only that (pass parameter this way) and leave the action signature intact, you'll still see the error. -
Sergio Tulentsev over 5 years(took the liberty to format some code. Horizontal scrolling on one-line snippets is horrible)
-
justinedps26 over 5 years@SergioTulentsev the error went away but i now have
undefined method
[]' for nil:NilClass` -
SteveTurczyn over 5 yearsThat's on line 7? Did you spell "params" correctly?
-
SteveTurczyn over 5 yearsI just realised you're not even using the argument! Just remove it from the
def add_item
line. -
SteveTurczyn over 5 years@SergioTulentsev Yes, my answer was to do both steps, I wasn't giving OP a choice. Good improvement on the formatting, I'll remember that for future.
-
SteveTurczyn over 5 yearsAnd which line is the error? Does it say
app/controllers/carts_controller.rb:8:in add_item
or `app/controllers/carts_controller.rb:9:in add_item' ? -
justinedps26 over 5 years@SteveTurczyn it says
app/controllers/carts_controller.rb:9:in
add_item' ` -
justinedps26 over 5 yearsLet us continue this discussion in chat.