Running a React app as a background process
Your Javascript file (distServer.js
) is your entry point – it's the file that you run to start your server. Your HTML file (index.html
) is only served as a response to the requests.
babel-node
is OK for development, but it's not suitable for production. You can precompile your Javascript files to vanilla Javascript, then use forever or pm2 as described in the question you already linked to in order to keep the server running even after you close your terminal.
How you organize your source files and compiled files is up to you, but here's one way to do it (quote from the documentation for an example Node server with Babel):
Getting ready for production use
So we've cheated a little bit by using
babel-node
. While this is great for getting something going. It's not a good idea to use it in production.We should be precompiling your files, so let's do that now.
First let's move our server
index.js
file tolib/index.js
.$ mv index.js lib/index.js
And update our
npm start
script to reflect the location change."scripts": { - "start": "nodemon index.js --exec babel-node --presets es2015,stage-2" + "start": "nodemon lib/index.js --exec babel-node --presets es2015,stage-2" }
Next let's add two new tasks
npm run build
andnpm run serve
."scripts": { "start": "nodemon lib/index.js --exec babel-node --presets es2015,stage-2", + "build": "babel lib -d dist --presets es2015,stage-2", + "serve": "node dist/index.js" }
Now we can use
npm run build
for precompiling our assets, andnpm run serve
for starting our server in production.$ npm run build $ npm run serve
This means we can quickly restart our server without waiting for
babel
to recompile our files.Oh let's not forget to add
dist
to our.gitignore
file.$ touch .gitignore dist
This will make sure we don't accidentally commit our built files to git.
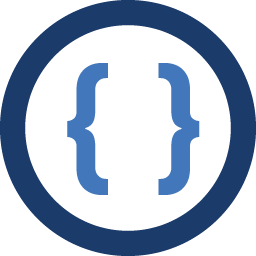
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm completely new to deploying front-end code and thus the question. I have a React App which I need to run as a background process, however I'm a little confused about the how to do this. I run a npm script
npm run build
to build, minify and serve the project on a server. The relevant code for the build process is this.
"prebuild": "npm-run-all clean-dist test lint build:html", "build": "babel-node tools/build.js", "postbuild": "babel-node tools/distServer.js"
This is the code inside the distServer.js
import express from 'express'; import path from 'path'; import open from 'open'; import compression from 'compression'; const port = 3000; const app = express(); app.use(compression()); app.use(express.static('dist')); app.get('*', function(req, res){ res.sendFile(path.join(__dirname, '../dist/index.html')); }); app.listen(port, function(err){ if(err){ console.log(err); }else{ open(`http://localhost:${port}`); } });
This works and the project runs, however the moment I close my terminal the project stops. The build process creates, three files,
index.html index.js styles.css
Now if I navigate to the index.html and open it in a browser, but naturally, nothing shows up. So I'm assuming that I'd have to run it as a node process. How do I do this on the production server and run it as a background process so that even if I exit the terminal the app continues to run. I have checked this issue, How to make a node.js application run permanently?
But this has a javascript file as the entry point, in my case it's a html file. I'm not sure how can I modify my scripts to run the front-end app permanently as a background process. Any help appreciated.