Save audio stream / Uint8List data in file flutter
I have added a WAVE/RIFF Header before writing my bytes into the file which gives the metadata to bytes and file.
I have 16 BIT PCM audio bytes with MONO channel in my stream. I am appending those bytes in a list of bytes when I am receiving the bytes.
List<int> recordedData = [];
recordedData.addAll(value);
Now I have all the recorded bytes on my list. After stopping my recorder I called the below function. Which takes all the data and sample rate for recorded. in my case its 44100.
await save(recordedData, 44100);
Future<void> save(List<int> data, int sampleRate) async {
File recordedFile = File("/storage/emulated/0/recordedFile.wav");
var channels = 1;
int byteRate = ((16 * sampleRate * channels) / 8).round();
var size = data.length;
var fileSize = size + 36;
Uint8List header = Uint8List.fromList([
// "RIFF"
82, 73, 70, 70,
fileSize & 0xff,
(fileSize >> 8) & 0xff,
(fileSize >> 16) & 0xff,
(fileSize >> 24) & 0xff,
// WAVE
87, 65, 86, 69,
// fmt
102, 109, 116, 32,
// fmt chunk size 16
16, 0, 0, 0,
// Type of format
1, 0,
// One channel
channels, 0,
// Sample rate
sampleRate & 0xff,
(sampleRate >> 8) & 0xff,
(sampleRate >> 16) & 0xff,
(sampleRate >> 24) & 0xff,
// Byte rate
byteRate & 0xff,
(byteRate >> 8) & 0xff,
(byteRate >> 16) & 0xff,
(byteRate >> 24) & 0xff,
// Uhm
((16 * channels) / 8).round(), 0,
// bitsize
16, 0,
// "data"
100, 97, 116, 97,
size & 0xff,
(size >> 8) & 0xff,
(size >> 16) & 0xff,
(size >> 24) & 0xff,
...data
]);
return recordedFile.writeAsBytesSync(header, flush: true);
}
And it's creating the playable WAV file.
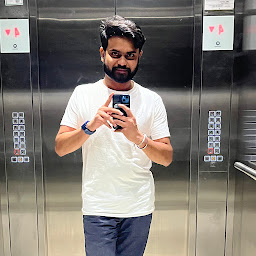
Comments
-
Dhalloo over 1 year
I am getting
Uint8List
from recorder plugin for Android and iOS both. I want to write the data in a local playable audio file whenever I am getting the data in my stream subscription of a mic. Is there any possible way to write the data?Currently, I am storing the data like
recordedFile.writeAsBytesSync(recordedData, flush: true);
It's writing data to file but not able to play from the file storage. But also if I read the same file and gives it's bytes to plugin it's playing the same buffer.
-
FilipeOS almost 4 yearsDid you managed to do this?
-
Dhalloo almost 4 years@FilipeOS Yes. I have to add some header and it's allowed me to write a playable audio file
-
Uttam Panchasara over 3 years@Dhalloo May I know which plugin are using, which is returning bytes?
-
Dhalloo over 3 years@UttamPanchasara I have used flutter sound plugin to fetch the bytes and play from the bytes.
-
who-aditya-nawandar over 2 years@Dhalloo Can you share the code please; how is flutter_sound returning bytes?
-
Dhalloo over 2 years@who-aditya-nawandar plugin is self-descriptive now. If you look into example
-
-
FilipeOS almost 4 yearsWhen I do
await save(_micChunks, 44100);
I getThe argument type List<Uint8List> can't be assigned to List<int>
. I'm using plugin sound_stream -
Dhalloo almost 4 yearsUint8List is extending to List<int> only. So in your case, as you have List<Uint8List> try with List<List<int>>
-
FilipeOS almost 4 yearsIf I change
List<int> recordedData = [];
toList<List<int>> data = _micChunks;
the last piece of code on header...data
say aList<int> cannot be assigned to int
-
Dhalloo almost 4 yearscan you share any link of the package or plugin that you used so I can guide you accordingly
-
FilipeOS almost 4 years
-
Dhalloo almost 4 yearsI am also using the same.
-
FilipeOS almost 4 yearsAnd can you help me? It's saving file but not playing at all.. Gives error github.com/CasperPas/flutter-sound-stream/issues/…
-
Dhalloo almost 4 years@FilipeOS how can I help you in this issue?
-
Uttam Panchasara over 3 years@Dhalloo I have same scenario as your only difference is I'm continually receiving audio bytes (real-time), I want to convert that raw bytes to text, any idea? Rate = 44100, channel = Mono
-
Ali Esfandiari about 3 yearsI recorded my audio by flutter webrtc, but after adding header I only receive noise.