save image to the gallery after taken from the camera
3,950
You can use package gallery_saver https://pub.dev/packages/gallery_saver
Get image with ImagePicker
and save with GallerySaver.saveImage
code snippet
void _takePhoto() async {
ImagePicker.pickImage(source: ImageSource.camera)
.then((File recordedImage) {
if (recordedImage != null && recordedImage.path != null) {
setState(() {
firstButtonText = 'saving in progress...';
});
GallerySaver.saveImage(recordedImage.path, albumName: albumName)
.then((bool success) {
setState(() {
firstButtonText = 'image saved!';
});
});
}
});
}
demo
full code
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:gallery_saver/gallery_saver.dart';
import 'package:image_picker/image_picker.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
String firstButtonText = 'Take photo';
String secondButtonText = 'Record video';
double textSize = 20;
String albumName ='Media';
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
color: Colors.white,
child: Column(
children: <Widget>[
Flexible(
flex: 1,
child: Container(
child: SizedBox.expand(
child: RaisedButton(
color: Colors.blue,
onPressed: _takePhoto,
child: Text(firstButtonText,
style:
TextStyle(fontSize: textSize, color: Colors.white)),
),
),
),
),
Flexible(
child: Container(
child: SizedBox.expand(
child: RaisedButton(
color: Colors.white,
onPressed: _recordVideo,
child: Text(secondButtonText,
style: TextStyle(
fontSize: textSize, color: Colors.blueGrey)),
),
)),
flex: 1,
)
],
),
),
));
}
void _takePhoto() async {
ImagePicker.pickImage(source: ImageSource.camera)
.then((File recordedImage) {
if (recordedImage != null && recordedImage.path != null) {
setState(() {
firstButtonText = 'saving in progress...';
});
GallerySaver.saveImage(recordedImage.path, albumName: albumName)
.then((bool success) {
setState(() {
firstButtonText = 'image saved!';
});
});
}
});
}
void _recordVideo() async {
ImagePicker.pickVideo(source: ImageSource.camera)
.then((File recordedVideo) {
if (recordedVideo != null && recordedVideo.path != null) {
setState(() {
secondButtonText = 'saving in progress...';
});
GallerySaver.saveVideo(recordedVideo.path, albumName: albumName)
.then((bool success) {
setState(() {
secondButtonText = 'video saved!';
});
});
}
});
}
// ignore: unused_element
void _saveNetworkVideo() async {
String path =
'https://sample-videos.com/video123/mp4/720/big_buck_bunny_720p_1mb.mp4';
GallerySaver.saveVideo(path, albumName: albumName).then((bool success) {
setState(() {
print('Video is saved');
});
});
}
// ignore: unused_element
void _saveNetworkImage() async {
String path =
'https://image.shutterstock.com/image-photo/montreal-canada-july-11-2019-600w-1450023539.jpg';
GallerySaver.saveImage(path, albumName: albumName).then((bool success) {
setState(() {
print('Image is saved');
});
});
}
}
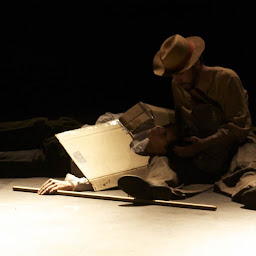
Comments
-
Giacomo M over 1 year
I use this code to take a picture with the plugin image_picker:
var image = await ImagePicker.pickImage(source: ImageSource.camera);
Now I would like to save the image in the gallery directory of the device.
According to the accepted answer to this post How to Save Image File in Flutter ? File selected using Image_picker plugin, it should have been done automatically, but I do not find in the gallery the picture taken.
I already added this permission to the manifest:
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
-
blackapps over 4 yearsIf you can pick an image file with an image picker then the image file is already on your device. Why would you make a copy of it?
-
blackapps over 4 years
I use this code to take a picture with the plugin image_picker:
To take a picture? Or to pick an image? Quite confusing. Is that opening the camera app? -
blackapps over 4 yearsIs this on Android Q?
-
blackapps over 4 yearsWhat is the value of image.path?
-
blackapps over 4 years
I would like to save the image in the gallery directory of the device
What do you consider to be the gallery directory of the device? The Gallery app shows images from all over your device. -
blackapps over 4 yearsIf you dont see the new image directly in the Gallery app then reboot your device.
-
Giacomo M over 4 years@blackapps I take a picture from the camera, but i am not able to save it to the gallery
-
blackapps over 4 yearsI still dont know what you mean by saving to the gallery but then you did not post any code. And you are not answering my questions.
-
Giacomo M over 4 years@blackapps I mean the gallery of the device, not in the folder of the app. For the path of the image I will answer to you tonight.
-
blackapps over 4 yearsWhat is the gallery of the device?
The Gallery app shows images from all over your device.
-
Giacomo M over 4 yearsIn android devices you have the "Gallery" with the images you have in the device.
-
blackapps over 4 yearsAs i already told you twice there is only a Gallery app which shows images from all over the device. The Gallery app is now often Fotos/Photos app from google. Now the question for the third time: "Where do you wanna store that image?".
-
Giacomo M over 4 years@blackapps these comments come to nothing, we can end. Thanks for your help
-
-
blackapps over 4 yearsWhat would be a/the value of
recordedImage.path
? -
chunhunghan over 4 yearsrecordedImage.path is the path image picker temporary save these files.
-
blackapps over 4 yearsYes. Please give an example of the value of such a path was the question. Where exactly is it stored?
-
chunhunghan over 4 yearsYou can print path. because in ios, android , emulator, they all different example is /data/0/emulated/your_app/cache
-
blackapps over 4 yearsThanks. I asked as i have no Flutter and @Giacomo M is strugling. I wonder if he is aware that there is a file already. He did not give the value for image.path.