Saving a text file on server using JavaScript
Solution 1
You must have a server-side script to handle your request, it can't be done using javascript.
To send raw data without URIencoding or escaping special characters to the php and save it as new txt
file you can send ajax request using post
method and FormData
like:
JS:
var data = new FormData();
data.append("data" , "the_text_you_want_to_save");
var xhr = (window.XMLHttpRequest) ? new XMLHttpRequest() : new activeXObject("Microsoft.XMLHTTP");
xhr.open( 'post', '/path/to/php', true );
xhr.send(data);
PHP:
if(!empty($_POST['data'])){
$data = $_POST['data'];
$fname = mktime() . ".txt";//generates random name
$file = fopen("upload/" .$fname, 'w');//creates new file
fwrite($file, $data);
fclose($file);
}
Edit:
As Florian mentioned below, the XHR fallback is not required since FormData
is not supported in older browsers (formdata browser compatibiltiy), so you can declare XHR variable as:
var xhr = new XMLHttpRequest();
Also please note that this works only for browsers that support FormData
such as IE +10.
Solution 2
It's not possible to save content to the website using only client-side scripting such as JavaScript and jQuery, but by submitting the data in an AJAX POST request you could perform the other half very easily on the server-side.
However, I would not recommend having raw content such as scripts so easily writeable to your hosting as this could easily be exploited. If you want to learn more about AJAX POST requests, you can read the jQuery API page:
http://api.jquery.com/jQuery.post/
And here are some things you ought to be aware of if you still want to save raw script files on your hosting. You have to be very careful with security if you are handling files like this!
File uploading (most of this applies if sending plain text too if javascript can choose the name of the file) http://www.developershome.com/wap/wapUpload/wap_upload.asp?page=security https://www.owasp.org/index.php/Unrestricted_File_Upload
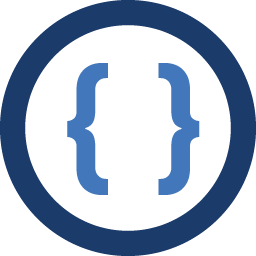
Admin
Updated on March 01, 2020Comments
-
Admin about 4 years
Is it possible to save text to a new text file using JavaScript/jQuery without using PHP? The text I'm trying to save may contain HTML entities, JS, HTML, CSS and PHP scripts that I don't want to escape or use urlencode!
If it's only can be achieved using PHP how can I pass the text to PHP without encoding it?