Scrollbar not stretching to fit the Text widget
Solution 1
In your question you're using pack
. pack
has options to tell it to grow or shrink in either or both the x and y axis. Vertical scrollbars should normally grow/shrink in the y axis, and horizontal ones in the x axis. Text widgets should usually fill in both directions.
For doing a text widget and scrollbar in a frame you would typically do something like this:
scr.pack(side="right", fill="y", expand=False)
text.pack(side="left", fill="both", expand=True)
The above says the following things:
- scrollbar is on the right (
side="right"
) - scrollbar should stretch to fill any extra space in the y axis (
fill="y"
) - the text widget is on the left (
side="left"
) - the text widget should stretch to fill any extra space in the x and y axis (
fill="both"
) - the text widget will expand to take up all remaining space in the containing frame (
expand=True
)
For more information see http://effbot.org/tkinterbook/pack.htm
Solution 2
Here is an example:
from Tkinter import *
root = Tk()
text = Text(root)
text.grid()
scrl = Scrollbar(root, command=text.yview)
text.config(yscrollcommand=scrl.set)
scrl.grid(row=0, column=1, sticky='ns')
root.mainloop()
this makes a text box and the sticky='ns'
makes the scrollbar go all the way up and down the window
Solution 3
Easy solution to use a textbox with an integrated scrollbar:
Python 3:
#Python 3
import tkinter
import tkinter.scrolledtext
tk = tkinter.Tk()
text = tkinter.scrolledtext.ScrolledText(tk)
text.pack()
tk.mainloop()
To read the textbox:
string = text.get("1.0","end") # reads from the beginning to the end
Of course you can shorten the imports if you want.
In Python 2 you import ScrolledText
instead.
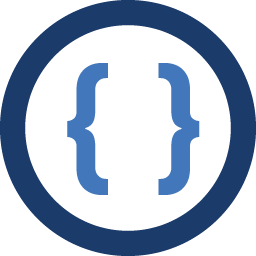
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I was able to get the
Scrollbar
to work with aText
widget, but for some reason it isn't stretching to fit the text box.Does anyone know of any way to change the height of the scrollbar widget or something to that effect?
txt = Text(frame, height=15, width=55) scr = Scrollbar(frame) scr.config(command=txt.yview) txt.config(yscrollcommand=scr.set) txt.pack(side=LEFT)
-
Russell Smith almost 11 yearsyour example neglects to set a weight to the row or column, so it probably won't resize properly.
-
Klamer Schutte about 9 yearsIn Python 2 this is:
from ScrolledText import ScrolledText
...
text = ScrolledTex(tk)
.