Sending UDP packages from ANDROID 2.2 (HTC desire)
12,355
I was having a very similar problem. My solution was to add:
uses-permission android:name="android.permission.INTERNET"
uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"
uses-permission android:name="android.permission.CHANGE_WIFI_MULTICAST_STATE"
to the Manifest.xml
file. Then I disabled all Windows firewall
and it worked. I was able to send a String
from my Droid
to a PC.
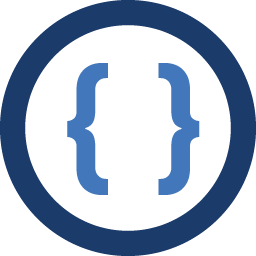
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
i have a lan and i want to send a upd message from my android (htc desire) to my PC. Theres a Wlan Router between them. The Problem is, that the UPD message never gets to the PC.
Code on the Android.:
package org.example.androidapp; import java.net.DatagramPacket; import java.net.DatagramSocket; import java.net.InetAddress; public class UDPClientAnd { public void sendUDPMessage(int port) throws java.io.IOException { DatagramSocket socket = new DatagramSocket(); InetAddress serverIP = InetAddress.getByName("192.168.1.110"); byte[] outData = ("Ping").getBytes(); DatagramPacket out = new DatagramPacket(outData,outData.length, serverIP,50005); socket.send(out); socket.close(); } }
I choose a high port on booth sides.
The Permissions on the Android are:
uses-permission android:name="android.permission.INTERNET" uses-permission android:name="android.permission.CHANGE_WIFI_MULTICAST_STATE"
The Server-Side is the PC just a simple programm for receiving:
package org.example.androidapp; import java.io.IOException; import java.net.DatagramPacket; import java.net.DatagramSocket; import java.net.InetAddress; import java.net.SocketException; public class UPDServerAnd implements Runnable{ public void run(){ byte[] inData = new byte[48]; byte[] outData = new byte[48]; String message; DatagramSocket socket; try { socket = new DatagramSocket(50005); while (true) { DatagramPacket in = new DatagramPacket(inData,inData.length); socket.receive(in); InetAddress senderIP = in.getAddress(); int senderPort = in.getPort(); message=new String(in.getData(),0,in.getLength()); System.out.println("Got "+message+" from "+senderIP+","+senderPort); outData = "Pong".getBytes(); DatagramPacket out = new DatagramPacket(outData,outData.length, senderIP,senderPort); socket.send(out); } } catch (SocketException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
So why is there no UDP Package visible coming from android? Even Wireshark only shows some ARP packages. Plz help :)