Showing an alert() dialog box if a form does not have valid values
Solution 1
On your form add something like this
<form name="frm1" onsubmit="InputChecker()">
Then in javascript
<script type="text/javascript">
function InputChecker()
{
if(document.getElementById({formElement}) != '') { // not empty
alert("This element needs data"); // Pop an alert
return false; // Prevent form from submitting
}
}
</script>
Also as others have said jQuery makes this a little bit easier. I highly recommend the jQuery Validate Plugin
Some people do find the alert box "annoying", so it may be better to append a message into the DOM to let the user know what needs to be fixed. This is useful if there are numerous errors as the errors will be more persistent allowing the user to see all the things they need to be fixed. Again, the jQuery Validate plugin has this functionality built in.
Solution 2
Attach an onsubmit
event to the form, and return false;
to stop the submission if checks fail.
Solution 3
Form validation with Javascript. Or easier with jQuery.
Basically, validate the form when the submit button is clicked (with an onsubmit handler), and then use an alert() box if needed. By the way, people usually hate alert boxes.
Solution 4
You have a number of options when it comes to client side validation. This is just one.
<form id="tehForm" method="post">
<input type="text" id="data2check" >
<input type="button" id="btnSubmit" />
</form>
<script type="text/javascript">
function submit_form(){
if(document.getElementById("data2check").value!="correct value"){
alert("this is wrong");
}else{
document.getElementById("tehForm").submit();
}
}
</script>
For a more indepth example check out this link
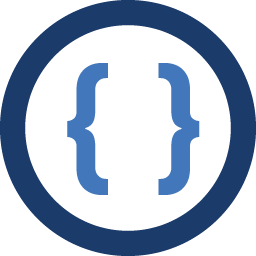
Admin
Updated on July 10, 2022Comments
-
Admin almost 2 years
I have a simple form which accepts a Title and a Contents variable from a textbox and a textarea. The form will send its data to a file called
add-post.php
. However, I am looking for a way to alert the user that either the textbox or the textarea has invalid data (is empty) in case they click the submission button.I was thinking that an
alert()
popup box would be the best idea because it doesn't redirect to any other page and the user never loses their data (imagine they entered a whole lot of text but forgot a title. Sending the data toadd-post.php
and performing the check there will result in loss of data for the user).However, I'm not sure how to actually implement the
alert()
popup. How would I make it so that the check is done AFTER they have clicked the submit button but BEFORE the data is sent off to the next file. Any advice is appreciated.