Showing or hiding elements based on variables in controller - Ionic
18,774
Solution 1
Add a ng-hide
directive to your button tag:
<button ng-hide=registered class="button button-clear button-block button-positive" ui-sref="register">
Register
</button>
In your JS file, declare this value in your $scope
to false
and set it to true
to hide the button:
app.controller('loginController', ['$scope', '$localstorage',
function($scope, $localstorage) {
$scope.registered = false;
// Check if the user has already requested a register, and if true, hide
// the 'Register' button
if ($localstorage.get("registrationRequested", false) === true) {
$scope.registered = true;
}
}
]);
Solution 2
do as following :
<button class="button button-clear button-block button-positive" ui-sref="register" ng-show='showBtn'>
Register
in controller :
app.controller('loginController', ['$scope', '$localstorage',
function($scope, $localstorage) {
if ($localstorage.get("registrationRequested", false) === true) {
$scope.showBtn = true;
}
else{
$scope.showBtn = false;
}
}]);
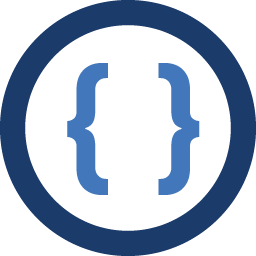
Author by
Admin
Updated on June 08, 2022Comments
-
Admin 12 months
For all I know this might be more an AngularJS issue than an Ionic specific one. I have a button in one of my views:
<button class="button button-clear button-block button-positive" ui-sref="register"> Register </button>
And in my controller I have this variable that I get from local storage that is either true or false and has to be hidden depending that the value is:
app.controller('loginController', ['$scope', '$localstorage', function($scope, $localstorage) { // Check if the user has already requested a register, and if true, hide // the 'Register' button if ($localstorage.get("registrationRequested", false) === true) { // How do I do this? } }]);
Now the first question probably is, is it even a best practice to manipulate the dom like that from my controller? And if not, where and how do I do it? If its' fine doing it in my controller, then how do I reference that button and hide it?
-
Admin almost 8 yearsWhoa! Thanks! As a quick question, is it safe to say that all the directives and features that are available in Angular is also available in Ionic? I'm kinda new to this as I'm sure you can tell..
-
MHX almost 8 yearsYes, that is safe to say.