Simple Number to Array with Individual Digits
Solution 1
Method number.toString().length() will return the number of digits. That is the same as the length of your needed array. Then you use your code as before, yet instead of printing you add the digit to the array.
int number = 1234567890;
int len = Integer.toString(number).length();
int[] iarray = new int[len];
for (int index = 0; index < len; index++) {
iarray[index] = number % 10;
number /= 10;
}
Solution 2
I would rather suggest you to use an ArrayList
, since to use an array, you would have to allocate the size in advance, for which you need to know the number of digits in your number, which you don't know.
So, either work with an array, and do the iteration over the number twice - once for finding size, and next for doing actual work. Else, move ahead with an ArrayList
.
Adding an element to an ArrayList
is quite simple. You just need to call - List#add(E)
method with appropriate parameter.
So, here's an extension of your solution: -
// Declare a List<Integer>, since it will store integers only.
List<Integer> digits = new ArrayList<Integer>():
int number = 1234567890;
while (number > 0) {
int digit = number % 10; // Store digit in a variable
number = number/10;
// Add digit to the list
digits.add(digit);
}
Alternatively, if you want to have only unique digits in your List
, then you should use a HashSet
, which automatically removes the duplicates.
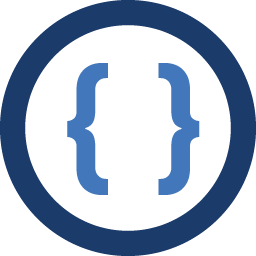
Admin
Updated on July 17, 2020Comments
-
Admin almost 4 years
I am exceptionally new to programming, but I am working on improving my skills as a programmer. Currently, I am working on a problem I gave myself where I am trying to take a variable number and make each of its digits a separate number in an array. I don't care about the order of the digits, so if they are reversed, then it doesn't matter to me. I know people have asked this question numerous times, but they always seem to use a lot of things that I don't understand. Since my school doesn't offer any Java courses, I only know what I have learned on my own, so if you could explain any terms you use in the code that aren't extremely trivial, that would be wonderful. Right now, I have written:
int number = 1234567890; while (number > 0) { System.out.println(number%10); number = number/10;
This works fine for printing the digits individually, but I can't figure out how to add them to the array. I greatly appreciate any help you can give, and please keep in mind that I much prefer simplicity over small size. Thank you in advance!
P.S. Some responses I've seen for similar questions include what I think are arrays of strings. In order for the part of the program that I have working to still work, I think that I need to use an array of integers. If you're curious, the rest of the code is used to determine if the numbers in the array are all different, in order to achieve the final result of determining if a number's digits are all distinct. It looks like this:
int repeats=0; int[] digitArray; digitArray = new int[10]; for (int i = 0; i < digitArray.length; i++) for (int j = 0; j < digitArray.length; j++) if ((i != j) && (digitArray[i]==digitArray[j])) unique = unique+1; System.out.println(unique==0);
-
Admin over 11 yearsWow! That was a quick reply. That is really helpful, too. My only question is, Why are you using "Integer" instead of "int", which is what I usually use?
-
Admin over 11 yearsThanks for the helpful and speedy reply! Do you think you could explain the HashSet a bit more? That function intrigues me, because it seems like it could potentially make my code much simpler.
-
Rohit Jain over 11 years@MatthewTyler.. You can work with an
int
too.Integer
is not necessarily required here. In fact, you should useint
wherever possible. And only useInteger
, where you can't manage without it, e.g. in a genericArrayList
declaration, where you can't have -ArrayList<int>
, but onlyArrayList<Integer>
-
Rohit Jain over 11 yearsSee the documentation - docs.oracle.com/javase/7/docs/api/java/util/HashSet.html . A HashSet is nothing but a
Set
, that stores just unique element. You knowsets
in mathematics right? It's just that. You can get more details in docs. -
Admin over 11 years@RohitJain.. I see. I've never heard of using Integer before, so I think I'll stick with int whenever possible.
-
Admin over 11 yearsI greatly appreciate all of the help you have thus far given, but if you could show me how I would implement the HashSet function into the actual code, then that would be absolutely superb. Thank you for your time.
-
Rohit Jain over 11 years@MatthewTyler.. Just replace the declaration of
ArrayList
with aHashSet
. It goes like this: -Set<Integer> digits = new HashSet<Integer>();
. And the rest is same. -
Admin over 11 yearsI get it now. Thanks for the information. I'll try that. Do you know why it comes up with an error whenever number is too large? My current code is as follows:
-
Rohit Jain over 11 years@MatthewTyler.. Because you can't store too large number in an
int
. Try usinglong
type for your original number. -
Admin over 11 yearsOh, I see. Thanks. I'm getting a warning to not use extended discussion in comments, so I'll stop bugging you now. You have been a tremendous help. Thank you, and have a wonderful day.
-
Max Dietz over 11 yearsI use Integer because int does not have a toString() method meaning you would have to use an ArrayList instead of a simple array.
-
Rohit Jain over 11 years@MaxDietz What this have to do with the
toString
method in Integer? And how will it affect the use ofint[]
instead ofInteger[]
? -
Rohit Jain over 11 years@MatthewTyler.. Did you have any issue with this solution?
-
Max Dietz over 11 yearsEdited to make a bit clearer. When initializing an array, which is a much simpler data structure than an ArrayList, you need to know its length beforehand, which is equal to the number of digits. To do so, casting to a string and counting the characters is the same as knowing the digits. Integer is an object that encapsulates an int, basically it stores an int but also has some methods, like toString, equals, etc.. It has a bit of bloat, and can be replaced by my edit, namely the static method
Integer.toString
as shown.