Socket connection over internet in Python?
Solution 1
Use this software to implement port-forwarding. I recommend you use another port for your server, say 5006, to prevent any problems related to using a very commonly used port like 80. Basically, the software works like this:
- You click Connect, and it searches for routers and if it finds yours, it lists the existing port mappings.
- You create a port mapping (on the right), default protocol is TCP
- You select a port on your router, say 5001 (called the External port)
- You select a port on your server, maybe 5006 (called the Internal port)
- The router will then be instructed to forward all data that arrives at port 5001 to your server, using your private IP, specifically to port 5006 on your server.
So all your client has to do is send data to the public IP of your server, specifically to port 5001. This data will of course first arrive at your router, which will behave as configured and forward everything to your server's port 5006. All this only works if your internet gateway supports port forwarding.
Client:
import socket
s = socket.socket()
host = '103.47.59.130'
port = 5001
s.connect((host, port))
while True:
try:
print "From Server: ", s.recv(1024)
s.send(raw_input("Client please type: "))
except:
break
s.close()
Server:
import socket
s = socket.socket() # Create a socket object
host = '192.168.0.104' #private ip address of machine running fedora
port = 5006
s.bind((host, port))
s.listen(5)
c, addr = s.accept()
print 'Got connection from', addr
while True:
c.send(raw_input("Server please type: "))
print "From Client: ", c.recv(1024)
c.close()
Solution 2
You need to use your public IP address or 0.0.0.0
in your server, and make sure your modem/router allows incoming connections at the specified port (usually called Port Forwarding).
Hope it helps!
Solution 3
Seems like you need to perform basic network troubleshooting. Your description says you can connect to other machines on your own network, but not a machine on another network. You might try the same sorts of tests on the machine on the other network: can it connect to other machines on its own network, can it connect to other machines off of its network.
Some tools which are very valuable include ping, tracepath, tcpdump, and nc (netcat).
Ultimately, if you can make the connection with netcat, you can assume the problem is with your code, but if you can't you can try and find the networking problem.
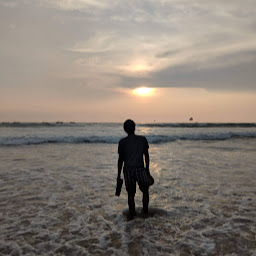
Sanyam Jain
Updated on February 14, 2020Comments
-
Sanyam Jain about 4 years
I have created a basic client server socket program in Python 2.7.x and it is running absolutely fine over the same network even on different machines but when I run server and client on different networks(server on my friend's network while client on mine) it does not return any error and keeps on waiting. I just can't understand how to debug the code. I am using port 80 by killing all the services on port 80. I have also done port forwarding on port 80 on both the machines.
My codes is as follows:
client.py
import socket s = socket.socket() host = '103.47.59.130' port = 80 s.connect((host, port)) while True: print "From Server: ", s.recv(1024) #This gets printed after sometime s.send(raw_input("Client please type: ")) s.close()
server.py
import socket s = socket.socket() # Create a socket object host = '192.168.0.104' #private ip address of machine running fedora port = 80 s.bind((host, port)) s.listen(5) c, addr = s.accept() print 'Got connection from', addr #this line never gets printed while True: c.send(raw_input("Server please type: ")) print "From Client: ", c.recv(1024) c.close()
It sometimes output **From Server: ** but doesnot send any message back and forth.
PS: I have searched on Stack Overflow earlier but I am unable to find anything relevant.
-
Sanyam Jain about 8 yearsDo I need to add public ip address in the server code? The IP address in the client.py is public only.
-
cdonts about 8 years@SanyamJain Yes. Using
192.168.0.104
will just listen for LAN connections. Use your public IP address or0.0.0.0
. -
tdelaney about 8 yearsThe server should use
0.0.0.0
so that the code can run on many machines, but if192.168.0.104
is in fact the server machine's address, it still works. The server is behind a NAT and as pointed out here, you need configure port-forwarding on the NAT to send port 80 to192.168.0.104
. Your friend's ISP may block incoming connection requests or maybe just 80 specifically, so experiment with others. -
Sanyam Jain about 8 yearsI have tried using 0.0.0.0 but Server still waiting for client to connect. I have change the port to 8009 also, do I need to do something else?
-
cdonts about 8 years@SanyamJain Did you open the port 8009 in your modem settings?
-
Sanyam Jain about 8 years@cdonts I have done port forwarding on port 8009 too.
-
Luke almost 8 yearsBy default, 5001 external will not go through to 5001 internal? But if you map the port you can make other ports go to other internal ports?
-
SoreDakeNoKoto almost 8 years@Luke Exactly. Your external ports dont map to internal ones, by default. You can make any external port map to any internal one; be sure to avoid ports below 1000.
-
Luke almost 8 yearsTrue, those ports are used by some services. I also found something hogging port 9000 which I thought was strange.
-
Prashant over 3 yearsIf I know the remote IP address why should I use port forwarding?
-
SoreDakeNoKoto over 3 years@Prashant Not exactly sure what you mean by remote IP address here. If the server you're trying to reach is outside your local network and behind NAT, then: (1) trying to reach it by its private/local address (usually 192.168.x.x.) is no use, since that address is...well, private. For local networks only, not used on the open web. (2) trying to reach it by its public (a.k.a. router) address is also no use, since you'd merely be addressing the server's router who has no idea to which local device it should route this totally-unknown incoming connection.
-
SoreDakeNoKoto over 3 yearsAnd this latter point is why you need port forwarding. To instruct the router, to direct all traffic arriving at one of its own ports to a specific port on your server.
-
Prashant over 3 years@SoreDakeNoKoto Suppose I know the remote/server address is 25.8.69.8, why should I need port forwarding?
-
SoreDakeNoKoto over 3 years@Prashant Like I said, if that address is public (and yours looks like it), then you don't need port forwarding. Simply put, if you can connect successfully to specific ports on that remote server from any device across the web (without any prior port forwarding), then you clearly don't need port forwarding -- at least for the actual functionality. Security is a different matter; it may not be the wisest thing to expose your server directly to the web that way, at least not without a decent firewall, but I'm no expert there.