Splitting a nested list into two lists
30,983
Solution 1
This is what the builtin function zip
is for.
my_list2, my_list1 = zip(*my_list)
If you want lists instead of tuples, you can do
my_list2, my_list1 = map(list, zip(*my_list))
Solution 2
You can use list comprehension.
my_list1 = [i[1] for i in my_list]
my_list2 = [i[0] for i in my_list]
Solution 3
using list comprehension
:
In [6]: lis1,lis2=[x[0] for x in my_list],[x[1] for x in my_list]
In [7]: lis1
Out[7]: [1320120000000L, 1320206400000L, 1320292800000L]
In [8]: lis2
Out[8]: [48596281, 48596281, 50447908]
or using operator.itemgetter()
:
In [19]: lis1,lis2=map(itemgetter(0),my_list) , map(itemgetter(1),my_list)
In [20]: lis1
Out[20]: [1320120000000L, 1320206400000L, 1320292800000L]
In [21]: lis2
Out[21]: [48596281, 48596281, 50447908]
timeit
comparisons:
In [42]: %timeit lis1,lis2=[x[0] for x in my_list],[x[1] for x in my_list] #winner if lists are required
100000 loops, best of 3: 1.72 us per loop
In [43]: %timeit my_list2, my_list1 = zip(*my_list) # winner if you want tuples
1000000 loops, best of 3: 1.62 us per loop
In [44]: %timeit my_list2, my_list1 = map(list, zip(*my_list))
100000 loops, best of 3: 4.58 us per loop
In [45]: %timeit lis1,lis2=map(itemgetter(0),my_list),map(itemgetter(1),my_list)
100000 loops, best of 3: 4.4 us per loop
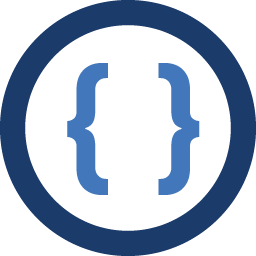
Author by
Admin
Updated on April 23, 2020Comments
-
Admin about 4 years
I have a nested list like this:
my_list = [[1320120000000, 48596281], [1320206400000, 48596281], [1320292800000, 50447908]]
I would like to split it into something that looks like this:
my_list1 = [48596281, 48596281, 50447908] my_list2 = [1320120000000, 1320206400000, 1320292800000]
I know that this is fairly simple, but I haven't been able to get it to work. Any help would be much appreciated.
-
kreativitea over 11 yearsfrom the python docs.
zip() in conjunction with the * operator can be used to unzip a list
-
-
Revolucion for Monica almost 7 yearsThanks for your answer, and how can I do when I don't know how many nested lists are in
*my_list
? -
Drachenfels over 6 yearsIn such case probably
my_lists = zip(*my_list)
later on by len(my_lists) you will learn how many lists you get in the end. -
Drachenfels over 6 yearswhat I found disturbing with
zip
is that even your nested list are perfectly symmetrical you may end up with messy data, for that reason list comprehension is probably slightly better, please consider:ab = [['a', 'A', 0], ['b', 1], ['c', 'C', 2]]; print(list(zip(*ab))); [('a', 'b', 'c'), ('A', 1, 'C')]
-
DJ_Stuffy_K about 6 yearsif each tuple has three elements [(a,b,c) (x,y,z)] and I want to extract (a,b) (x,y) in one place and (c,z) in another place. how to proceed please tell.