Python: loop over a list of string and using split()
11,527
Solution 1
Don't use split()
here as it'll also strip the trailing '\n'
, use split(' ')
.
>>> text = ['James Fennimore Cooper\n', 'Peter, Paul, and Mary\n',
... 'James Gosling\n']
>>> [y for x in text for y in x.split(' ')]
['James', 'Fennimore', 'Cooper\n', 'Peter,', 'Paul,', 'and', 'Mary\n', 'James', 'Gosling\n']
And in case the number of spaces are not consistent then you may have to use regex:
import re
[y for x in text for y in re.split(r' +', x)]]
Solution 2
Building on @Aशwini चhaudhary's response, if you're interested in removing the trailing ,
s and \n
s from your string fragments, you could do
[y.rstrip(',\n') for x in text for y in x.split(' ')]
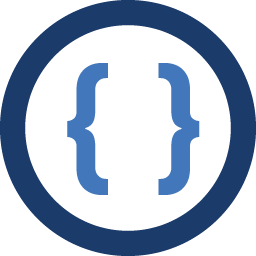
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to split the elements of a list:
text = ['James Fennimore Cooper\n', 'Peter, Paul, and Mary\n', 'James Gosling\n'] newlist = ['James', 'Fennimore', 'Cooper\n', 'Peter', 'Paul,', 'and', 'Mary\n', 'James', 'Gosling\n']
My code so far is:
newlist = [] for item in text: newlist.extend(item.split()) return newlist
And I get the error:
builtins.AttributeError: 'list' object has no attribute 'split'