Python: if list contains string print all the indexes / elements in the list that contain it
Solution 1
Try this:
list = data_array
string = str(raw_input("Search keyword: "))
print string
for s in list:
if string in str(s):
print 'Yes'
print list.index(s)
Editted to working example. If you only want the first matching index you can also break after the if statement evaluates true
Solution 2
matches = [s for s in my_list if my_string in str(s)]
or
matches = filter(lambda s: my_string in str(s), my_list)
Note that 'nice' in 3456
will raise a TypeError
, which is why I used str()
on the list elements. Whether that's appropriate depends on if you want to consider '45'
to be in 3456
or not.
Solution 3
print filter(lambda s: k in str(s), l)
Solution 4
To print all the elements that contains nice
mylist = ['nice1', 'def456', 'ghi789', 'nice2', 'nice3']
sub = 'nice'
print("\n".join([e for e in mylist if sub in e]))
>>> nice1
nice2
nice3
To get the index of elements that contain nice (irrespective of the letter case)
mylist = ['nice1', 'def456', 'ghi789', 'Nice2', 'NicE3']
sub = 'nice'
index_list = []
i = 0
for e in mylist:
if sub in e.lower():
index_list.append(i)
i +=1
print(index_list)
>>> [0, 3, 4]
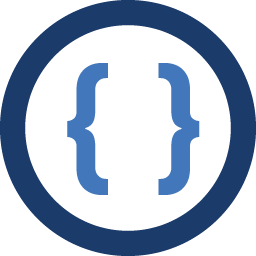
Admin
Updated on July 16, 2020Comments
-
Admin almost 4 years
I am able to detect matches but unable to locate where are they.
Given the following list:
['A second goldfish is nice and all', 3456, 'test nice']
I need to search for match (i.e. "nice") and print all the list elements that contain it. Ideally if the keyword to search were "nice" the results should be:
'A second goldfish is nice and all' 'test nice'
I have:
list = data_array string = str(raw_input("Search keyword: ")) print string if any(string in s for s in list): print "Yes"
So it finds the match and prints both, the keyword and "Yes" but it doesn't tell me where it is.
Should I iterate through every index in list and for each iteration search "string in s" or there is an easier way to do this?