SQL Server trigger with loop for multiple row insertion
11,280
If you cannot change the stored procedure, then this might be one of the (very few) cases when a cursor comes to the rescue. Double loops, in fact:
ALTER TRIGGER [dbo].[ConferenceDayTrigger]
ON [dbo].[Conferences]
AFTER INSERT
AS
BEGIN
DECLARE @ID INT;
DECLARE @dayC INT;
DECLARE @counter INT
SET @counter = 1;
DECLARE yucky_Cursor CURSOR FOR
SELECT IDConference, DATEDIFF(DAY, start,finish) FROM Inserted;
OPEN yucky_Cursor; /*Open cursor for reading*/
FETCH NEXT FROM yucky_Cursor INTO @ID, @dayC;
WHILE @@FETCH_STATUS = 0
BEGIN
WHILE @counter <= @dayC + 1
BEGIN
EXEC AddConferenceDay @Id, @counter;
SET @counter = @counter + 1;
END;
FETCH NEXT FROM yucky_Cursor INTO @ID, @dayC;
END;
CLOSE yucky_Cursor;
DEALLOCATE yucky_Cursor;
END;
I suspect there is a way to refactor and get rid of the cursor and use set-based operations.
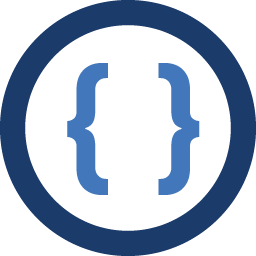
Author by
Admin
Updated on June 25, 2022Comments
-
Admin about 2 years
I've created trigger for my database which handles some insertion but when I add multiple values in 1 SQL query it doesn't work:
ALTER TRIGGER [dbo].[ConferenceDayTrigger] ON [dbo].[Conferences] AFTER INSERT AS BEGIN DECLARE @ID INT DECLARE @dayC INT DECLARE @counter INT SET @counter = 1 SET @ID = (SELECT IDConference FROM Inserted) SET @dayC = (SELECT DATEDIFF(DAY, start,finish) FROM Inserted) WHILE @counter <= @dayC + 1 BEGIN EXEC AddConferenceDay @Id, @counter SET @counter = @counter +1 END END
For single insertion it works ok. But what should I change/add to make it execute for each row of inserted values?