Struct Constructor in C++?
Solution 1
In C++ the only difference between a class
and a struct
is that members and base classes are private by default in classes, whereas they are public by default in structs.
So structs can have constructors, and the syntax is the same as for classes.
Solution 2
struct TestStruct {
int id;
TestStruct() : id(42)
{
}
};
Solution 3
All the above answers technically answer the asker's question, but just thought I'd point out a case where you might encounter problems.
If you declare your struct like this:
typedef struct{
int x;
foo(){};
} foo;
You will have problems trying to declare a constructor. This is of course because you haven't actually declared a struct named "foo", you've created an anonymous struct and assigned it the alias "foo". This also means you will not be able to use "foo" with a scoping operator in a cpp file:
foo.h:
typedef struct{
int x;
void myFunc(int y);
} foo;
foo.cpp:
//<-- This will not work because the struct "foo" was never declared.
void foo::myFunc(int y)
{
//do something...
}
To fix this, you must either do this:
struct foo{
int x;
foo(){};
};
or this:
typedef struct foo{
int x;
foo(){};
} foo;
Where the latter creates a struct called "foo" and gives it the alias "foo" so you don't have to use the struct
keyword when referencing it.
Solution 4
Yes, but if you have your structure in a union then you cannot. It is the same as a class.
struct Example
{
unsigned int mTest;
Example()
{
}
};
Unions will not allow constructors in the structs. You can make a constructor on the union though. This question relates to non-trivial constructors in unions.
Solution 5
Class, Structure and Union is described in below table in short.
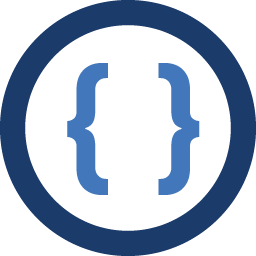
Admin
Updated on August 23, 2021Comments
-
Admin over 2 years
Can a
struct
have a constructor in C++?I have been trying to solve this problem but I am not getting the syntax.