Styling of initial default value of a text field
Solution 1
If you want to style only the *
, you're out of luck. No way to do that in just CSS. There is, however, way of doing this with altering your DOM:
<div class="input-container">
<input type="text" name="fName" class="inpField" size="20" value="First Name" />
<span class="input-addon">*</span>
</div>
Then, the CSS:
.input-container {
position: relative;
display: inline-block;
}
.input-addon {
position: absolute;
right: 10px;
top: 5px;
color: red;
}
Solution 2
You can target input
with the specified value of thevalue
attribute (if you want to target value
starting with "First Name", you can use ^=
instead of =
):
input[type="text"][value="First Name"] {
font-size: 10px;
color: red;
<...>
}
Solution 3
Nowadays you can do this:
<input type="email"
name="email"
placeholder="Your Email"
id="newsletter-subscribe-email-field">
Then style it like:
#newsletter-subscribe-email-field::placeholder,
#newsletter-subscribe-email-field::-webkit-input-placeholder,
#newsletter-subscribe-email-field:-ms-input-placeholder,
#newsletter-subscribe-email-field::-ms-input-placeholder {
color:#5472e8;
}
::placeholder CSS pseudo-element
Solution 4
Simply doing this will suffice:
input[value] {
}
You can also prepend the selector with a PARENT id / class name to ensure it's unique for each form if need be.
http://jsfiddle.net/samuidavid/vz9qxsfw/
Solution 5
you can use this ::
text.inpField {
font-size: 10px;
color: red;
}
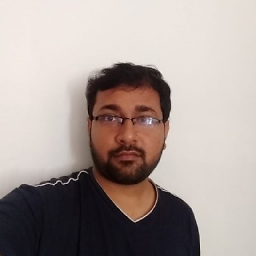
Probosckie
Hi, I am a javascript programmer and I like everything about frontend and ReactJS
Updated on June 04, 2022Comments
-
Probosckie 7 months
I have a html input textfield as follows:
<input type="text" name="fName" class="inpField" size="20" value="First Name *" />
Now I want to do selective styling of some text as follows:
I have the default value set as "First Name *". Using css- I want to apply a global style to that whole thing- such as font-family, font-size.
Then I want to selectively style the asterisk (*) after the First Name with color red. Since that * is a value contained in an attribute- how do I selectively select only the asterisk part in the value attribute to make it red.
Please help.
-
David Wilkinson over 7 yearsCertainly correct, but what if the content in the VALUE for the field changes in the future? You'd have to update the value="" CSS rule accordingly to match. Perhaps safer to target a parent id / class and input[value]?
-
potashin over 7 years@samuidavid: well,
id
is more flexible of course, but the question is about targeting specifiedvalue
, so I'm sticking to the answer. -
Probosckie over 7 yearsI have the default value set as "First Name ". Using css- I want to apply a global style to that whole thing- such as font-family, font-size. Then I want to selectively style the asterisk () after the First Name with color red. Since that * is a value contained in an attribute- how do I selectively select only the asterisk part in the value attribute to make it red.
-
Probosckie over 7 yearsI have the default value set as "First Name ". Using css- I want to apply a global style to that whole thing- such as font-family, font-size. Then I want to selectively style the asterisk () after the First Name with color red. Since that * is a value contained in an attribute- how do I selectively select only the asterisk part in the value attribute to make it red.
-
potashin over 7 years@Prabhas : I don't think it is possible in pure CSS