Swift Custom Fonts Xcode
Solution 1
These are the steps to add a custom font to you application:
- Add "gameOver.ttf" font in your application ( Make sure that it's included in the target)
- Modify the application-info.plist file.
- Add the key "Fonts provided by application" in a new row
- and add "gameOver.ttf" as new item in the Array "Fonts provided by application".
Now the font will be available in Interface Builder. To use the custom font in code we need to refer to it by name, but the name often isn’t the same as the font’s filename
There are 2 ways to find the name:
- Install the font on your Mac. Open Font Book, open the font and see what name is listed.
- Programmatically list the available fonts in your app
for the second approach add this line is your app delegate’s didFinishLaunchingWithOptions
print(UIFont.familyNames)
To list the fonts included in each font family, in Swift 5:
func printFonts() {
for familyName in UIFont.familyNames {
print("\n-- \(familyName) \n")
for fontName in UIFont.fontNames(forFamilyName: familyName) {
print(fontName)
}
}
}
after finding the name of your custom font you may use it like this:
SKLabelNode(fontNamed: "gameOver") // put here the correct font name
or in a simple label :
cell.textLabel?.font = UIFont(name: "gameOver", size: 16) // put here the correct font name
Solution 2
Swift 4 and 5. I have created an enum for App fonts. First install fonts on system by double click on desired font. Then installed font will appear under Custom fonts in Attribute inspector.
import Foundation
import UIKit
private let familyName = "Montserrat"
enum AppFont: String {
case light = "Light"
case regular = "Regular"
case bold = "Bold"
func size(_ size: CGFloat) -> UIFont {
if let font = UIFont(name: fullFontName, size: size + 1.0) {
return font
}
fatalError("Font '\(fullFontName)' does not exist.")
}
fileprivate var fullFontName: String {
return rawValue.isEmpty ? familyName : familyName + "-" + rawValue
}
}
Usage
self.titleLabel?.font = AppFont.regular.size(12.0)
Solution 3
Along with the above answer, I would like to add an another answer on installing the custom fonts to Xcode which can be access to Mac as well as MAC.
1. Select and download your favourite font styles from here
2. Unzip the download folder and select all the fonts from folder and double tap to open
3. Pop up appears to select all the fonts > check all and install
4. Open Xcode, one can see from Attribute inspector for selected label
That's all :)
Watch out the attached pic for more ref:
Font Lato appeared in Xcode workspace
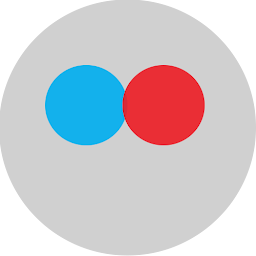
theenigma
Updated on September 05, 2021Comments
-
theenigma over 2 years
I am creating a game in Xcode (Version 7.0 Beta) using Swift, and I would like to display the label "Game Over" at the end of the game in the font "gameOver.ttf". I have added the font to my resources folder. I do not know how to reference it in my code. Can I please get help? My Code:
let label = SKLabelNode(fontNamed: "gameOver") label.text = "Game Over" label.fontColor = SKColor.redColor() label.fontSize = 150 label.position = CGPointMake(0, 100) label.horizontalAlignmentMode = .Center node.addChild(label)
-
marika.daboja about 3 yearsOMG..that was my missing link - I didn't add my custom font to the Info.plist Thank you! You made my day!