Swift Error: Cannot pass immutable value as inout argument: 'pChData' is a 'let' constant
Solution 1
You are trying to access/modify pChData
argument, which you can't unless or until you declare it as inout
parameter. Learn more about inout
parameter here. So try with the following code.
func receivedData(inout pChData: UInt8, andLength len: CInt) {
var receivedData: Byte = Byte()
var receivedDataLength: CInt = 0
memcpy(&receivedData, &pChData, Int(len));
receivedDataLength = len
AudioHandler.sharedInstance.receiverAudio(&receivedData, WithLen: receivedDataLength)
}
Solution 2
Arguments passed to a function are immutable inside the function by default.
You need to make a variable copy (Swift 3 compatible):
func receivedData(pChData: UInt8, andLength len: CInt) {
var pChData = pChData
var receivedData: Byte = Byte()
var receivedDataLength: CInt = 0
memcpy(&receivedData, &pChData, Int(len)); // Getting the error here
receivedDataLength = len
AudioHandler.sharedInstance.receiverAudio(&receivedData, WithLen: receivedDataLength)
}
or, with Swift 2, you can add var
to the argument:
func receivedData(var pChData: UInt8, andLength len: CInt) {
var receivedData: Byte = Byte()
var receivedDataLength: CInt = 0
memcpy(&receivedData, &pChData, Int(len)); // Getting the error here
receivedDataLength = len
AudioHandler.sharedInstance.receiverAudio(&receivedData, WithLen: receivedDataLength)
}
Third option, but that's not what you're asking for: make the argument an inout. But it will also mutate pchData outside of the func, so it looks like you don't want this here - this is not in your question (but I could have read that wrong of course).
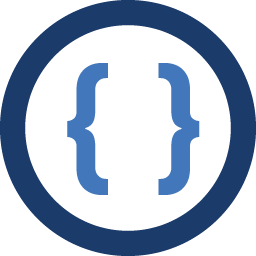
Admin
Updated on July 22, 2022Comments
-
Admin almost 2 years
I have a function that looks like the following:
func receivedData(pChData: UInt8, andLength len: CInt) { var receivedData: Byte = Byte() var receivedDataLength: CInt = 0 memcpy(&receivedData, &pChData, Int(len)); // Getting the error here receivedDataLength = len AudioHandler.sharedInstance.receiverAudio(&receivedData, WithLen: receivedDataLength) }
Getting the error:
Cannot pass immutable value as inout argument: 'pChData' is a 'let' constant
Though none of the arguments which I am passing here are
let
constants. Why am I getting this?