to_d to always return 2 decimals places in ruby
Solution 1
In addition to mcfinnigan's answer, you can also use the following to get 2 decimal places
'%.2f' % 500 # "500.00"
This use case is known as the string format operator
Solution 2
Since you are using Rails and this seems to be related to a view, there's number_with_precision
:
number_with_precision(500, precision: 2)
#=> "500.00"
I18n.locale = :de
number_with_precision(500, precision: 2)
#=> "500,00"
For currencies I'd suggest number_to_currency
:
number_to_currency(500)
#=> "$500.00"
Solution 3
Here's a hint. 500.00 is a representation of the number 500.0
Specifically, sprintf will help you:
irb(main):004:0> sprintf "%.2f", 500.0
=> "500.00"
Solution 4
Do not use floating point numbers to represent money. See this question for a good overview of why this is a bad idea.
Instead, store monetary values as integers (representing cents), or have a look at the money gem that provides lots of useful functionality for dealing with such values.
Solution 5
There was a requirement to round DOWN.
Most other answers round 500.016 UP to 500.02
Try:
def self.round_down(x, n = 2)
"%.#{n}f" % x.to_d.truncate(n)
end
irb(main):024:0> x=500.0; '%.2f' % x.to_d.truncate(2)
=> "500.00"
irb(main):025:0> x=500.016; '%.2f' % x.to_d.truncate(2)
=> "500.01"
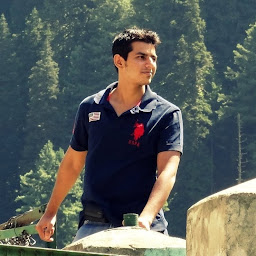
Varun Singh
Updated on September 20, 2020Comments
-
Varun Singh over 3 years
I'm dealing with currencies and I want to round down the number to 2 decimal places. Even if the number is 500.0, I would like it to be 500.00 to be consistent. When I do "500.00".to_d it converts it to 500.0.
Whats a good way of changing this behavior? I also use this method to round down to 2 digits and make sure it always has 2 decimals.
def self.round_down(x, n=2) s = x.to_s l = s.index('.') ? s.index('.') + 1 + n : s.length s = s[0, l] s = s.index('.') ? s.length - (s.index('.') + 1) == 1 ? s << '0' : s : s << '.00' s.to_f end
-
Camille Goudeseune about 10 yearsAs the proverb goes, Real Programmers can write C in any language!
'%.2f' % 500
is the Ruby idiom. -
mcfinnigan about 10 years@CamilleGoudeseune I blame it on far too many years of Java :/
-
Lev Lukomsky about 8 yearssprintf is more explicit than % and I can easily get help about formatting parameters by pressing F1 on sprintf in my IDE
-
webaholik over 5 yearsCaveat: this will round down or UP, eg. 500.016 becomes 500.02
-
webaholik over 5 yearsCaveat: this will round down or UP, eg. 500.016 becomes 500.02
-
jvnill over 5 yearsyep expected behavior would be to follow the rules for rounding numbers
-
ricks almost 3 yearsJust want to add that this answer covers a nil value, its great for
.erb
files.