Transparent card but with shadow effect from elevation
925
Hii Christophorus Anindityo N
Make a class for BoxShadow property of container.
class CustomBoxShadow extends BoxShadow {
final BlurStyle blurStyle;
const CustomBoxShadow({
Color color = const Color(0xFF000000),
Offset offset = Offset.zero,
double blurRadius = 0.0,
this.blurStyle = BlurStyle.normal,
}) : super(color: color, offset: offset, blurRadius: blurRadius);
@override
Paint toPaint() {
final Paint result = Paint()
..color = color
..maskFilter = MaskFilter.blur(this.blurStyle, blurSigma);
assert(() {
if (debugDisableShadows)
result.maskFilter = null;
return true;
}());
return result;
}
}
And use this class in a Container
Container(
child: Center(
child: Container(
height: 200.0,
width: 300.0
decoration: BoxDecoration(
boxShadow: [
CustomBoxShadow(
color: Colors.black,
offset: Offset(5.0, 5.0),
blurRadius: 5.0,
blurStyle: BlurStyle.outer
)
],
),
child: Text("Transparent Card with Shadow", style:TextStyle(fontSize:15))),
)
)
Now you are good to code :)
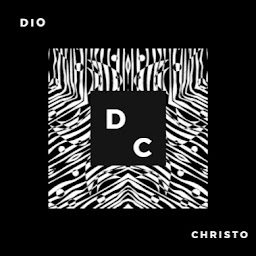
Author by
Christophorus Anindityo N
Updated on December 27, 2022Comments
-
Christophorus Anindityo N over 1 year
I'm having trouble making a card that has a transparent white color (opacity 0.4). But, with shadow from the elevation effect.
If I remove the elevation, there's no shadow effect and the card look transparent. But, if I add some elevation, the transparent effect ruined. Here's what I've tried:
Widget cardMenu(String title) { return Container( padding: EdgeInsets.symmetric(horizontal: UIComponent.componentPadding), child: Stack( alignment: Alignment.topCenter, children: [ Positioned( top: -100, child: Container( child: Container( height: 200, width: 200, decoration: BoxDecoration( color: Colors.white, shape: BoxShape.circle, ), child: Center( child: Text( title, style: TextStyle(color: Colors.transparent), ), ), ), ) ), Align( alignment: Alignment.bottomCenter, child: Card( elevation: 0, color: Colors.white.withOpacity(0.4), shape: RoundedRectangleBorder( borderRadius: BorderRadius.all( Radius.circular(UIComponent.cardButtonRadius), ), ), child: Container( height: 350, width: 180, child: Column( mainAxisSize: MainAxisSize.min, mainAxisAlignment: MainAxisAlignment.end, children: [ Container( padding: EdgeInsets.all(UIComponent.widgetPadding), child: Text( title, style: TextStyle( fontWeight: FontWeight.bold, fontSize: UIComponent.h1, color: UIComponent.neutralDark, ), ), ) ], ), ), ), ) ], ), );
}
The output of my code:
What I'm expecting to be:
-
Christophorus Anindityo N about 3 yearsHi!, thanks for answered my question. I tried your code above, but it didnt have rounded border like the card i was questioning. Can you make it to have the shape of rounded border?
-
Hardik Khatri about 3 yearsadd " borderRadius: BorderRadius.circular(10)" in decoration property and you are good to go.