TTransportException Exception while working with Apache Thrift
When you use TNonblockingServerSocket
, you need to use TFramedTransport
both server- and client-side. The documentation of TNonblockingServerSocket
is quite explicit about that:
To use this server, you MUST use a TFramedTransport at the outermost transport, otherwise this server will be unable to determine when a whole method call has been read off the wire. Clients must also use TFramedTransport.
Your client should therefore look like this:
TTransport transport;
try {
transport = new TSocket("localhost", Config.THRIFT_PORT);
transport.open();
TProtocol protocol = new TBinaryProtocol(new TFramedTransport(transport));
PringService.Client client = new PringService.Client(protocol);
String result = client.importPringer(2558456, true);
System.out.println("Result String is ::"+result);
transport.close();
} catch (TTransportException e) {
e.printStackTrace();
} catch (TException e) {
e.printStackTrace();
}
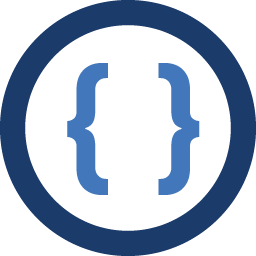
Admin
Updated on June 14, 2022Comments
-
Admin about 2 years
I am working with apache Thrift. I am getting TTransportException exception while everthing looks fine with my code. Here is my Thrift Server Code:
private TNonblockingServerSocket socket; /** * @breif Store processor instance. */ private PringService.Processor processor; /** * Store server instance. */ private TServer tServer; /** * * @breif A handle to the unique Singleton instance. */ static private ThriftServer _instance = null; /** * @breif The unique instance of this class. * @throws TTransportException */ static public ThriftServer getInstance() throws TTransportException { if (null == _instance) { _instance = new ThriftServer(); } return _instance; } /** * @breif A Ctor for ThriftServer. Initialize all members. * @throws TTransportException */ private ThriftServer() throws TTransportException { socket = new TNonblockingServerSocket(Config.THRIFT_PORT); processor = new PringService.Processor(new Handler()); THsHaServer.Args args = new THsHaServer.Args(socket); args.processor(processor); args.transportFactory(new TFramedTransport.Factory()); args.inputProtocolFactory(new TBinaryProtocol.Factory()); args.outputProtocolFactory(new TBinaryProtocol.Factory()); tServer = new THsHaServer(args); /*tServer = new THsHaServer(processor, socket, new TFramedTransport.Factory(), new TFramedTransport.Factory(), new TBinaryProtocol.Factory(), new TBinaryProtocol.Factory());*/ } /** * @breif main method * @param args the command line arguments * @throws TTransportException */ public static void main(String[] args) throws TTransportException { // To Run it directly from PringCore.jar, else use SmsProcessor Helper functionality ThriftServer server = new ThriftServer(); server.execute(args); } @Override /** * @breif Starts the execution. */ protected void execute(String[] args) { if (db != null) { db.close(); } tServer.serve(); }
private static class Handler implements PringService.Iface { ...... } }
And This is my thrift client:
TTransport transport; try { transport = new TSocket("localhost", Config.THRIFT_PORT); transport.open(); TProtocol protocol = new TBinaryProtocol(transport); PringService.Client client = new PringService.Client(protocol); String result = client.importPringer(2558456, true); System.out.println("Result String is ::"+result); transport.close(); } catch (TTransportException e) { e.printStackTrace(); } catch (TException e) { e.printStackTrace(); }
When I run my Thrift server and then run thrift client, I get the following exception:
org.apache.thrift.transport.TTransportException at org.apache.thrift.transport.TIOStreamTransport.read(TIOStreamTransport.java:132) at org.apache.thrift.transport.TTransport.readAll(TTransport.java:84) at org.apache.thrift.protocol.TBinaryProtocol.readAll(TBinaryProtocol.java:378) at org.apache.thrift.protocol.TBinaryProtocol.readI32(TBinaryProtocol.java:297) at org.apache.thrift.protocol.TBinaryProtocol.readMessageBegin(TBinaryProtocol.java:204) at org.apache.thrift.TServiceClient.receiveBase(TServiceClient.java:69)
Am I using mis-matching transport sockt/layer on my thrift server or client ? Or there is something other wrong?
Thanks in Advance for your guidance :)