Uncaught ReferenceError: require is not defined on karma start karma.conf.js
Solution 1
Browser don't understand require so you need to pre-process your files before you serve them to the browser.You can config webpack into karma.config so karma can use webpack to preprocess your files before testing. You also need karma webpack install with,
npm i --save-dev karma-webpack
There are many ways to do this, i did this way.
var path = require('path');
var webpackConfig = require('./webpack.config');
var entry = path.resolve(webpackConfig.context, webpackConfig.entry);
var preprocessors = {};
preprocessors[entry] = ['webpack'];
module.exports = function(config) {
config.set({
// base path that will be used to resolve all patterns (eg. files, exclude)
basePath: '',
// frameworks to use
// available frameworks: https://npmjs.org/browse/keyword/karma-adapter
frameworks: ['chai','mocha'],
// list of files / patterns to load in the browser
files: [entry],
webpack:webpackConfig,
// list of files to exclude
exclude: [
],
// preprocess matching files before serving them to the browser
// available preprocessors: https://npmjs.org/browse/keyword/karma-preprocessor
preprocessors:preprocessors,
// test results reporter to use
// possible values: 'dots', 'progress'
// available reporters: https://npmjs.org/browse/keyword/karma-reporter
reporters: ['progress'],
// web server port
port: 9876,
// enable / disable colors in the output (reporters and logs)
colors: true,
// level of logging
// possible values: config.LOG_DISABLE || config.LOG_ERROR || config.LOG_WARN || config.LOG_INFO || config.LOG_DEBUG
logLevel: config.LOG_INFO,
// enable / disable watching file and executing tests whenever any file changes
autoWatch: true,
// start these browsers
// available browser launchers: https://npmjs.org/browse/keyword/karma-launcher
browsers: ['Chrome'],
// Continuous Integration mode
// if true, Karma captures browsers, runs the tests and exits
singleRun: false,
// Concurrency level
// how many browser should be started simultanous
concurrency: Infinity,
plugins:[
require('karma-webpack'),
('karma-chai'),
('karma-mocha'),
('karma-chrome-launcher')
]
})
}
here is a seed i worked on with karma, webpack, angularjs.
take a look and good luck.
Solution 2
Follow this guide to set up Karma if you are using require to load your modules:
https://karma-runner.github.io/0.8/plus/RequireJS.html
or if you are using browserify for bundling, give this a try:
https://github.com/nikku/karma-browserify
you may also wanna look at this question: How to test browserify project using karma/jasmine
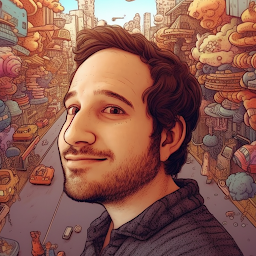
Comments
-
Adam Weitzman about 2 years
Using Karma and Jasmine for unit testing on the angular front-end of a rails app. It appears I've done everything known to man to get through this error and I'm left with a million dependencies in my package.json. Here is my Karma.conf.js:
module.exports = function(config) { config.set({ // base path that will be used to resolve all patterns (eg. files, exclude) basePath: '', // frameworks to use // available frameworks: https://npmjs.org/browse/keyword/karma-adapter // list of files / patterns to load in the browser files: [ //angular mocks 'bower_components/angular/angular.js', 'bower_components/angular-mocs/angular-mocks.js', 'bower_components/angular-resource/angular-resource.js', //load modules 'public/app/app.js', //test file locations 'app/**/*.js', 'spec/**/*.js', 'public/**/*.js' ], // list of files to exclude exclude: [ ], // preprocess matching files before serving them to the browser // available preprocessors: https://npmjs.org/browse/keyword/karma-preprocessor preprocessors: { }, // test results reporter to use // possible values: 'dots', 'progress' // available reporters: https://npmjs.org/browse/keyword/karma-reporter reporters: ['progress'], // web server port port: 9876, // enable / disable colors in the output (reporters and logs) colors: true, // level of logging // possible values: config.LOG_DISABLE || config.LOG_ERROR || config.LOG_WARN || config.LOG_INFO || config.LOG_DEBUG logLevel: config.LOG_INFO, // enable / disable watching file and executing tests whenever any file changes autoWatch: true, // start these browsers // available browser launchers: https://npmjs.org/browse/keyword/karma-launcher browsers: ['Chrome', 'Firefox'], // Continuous Integration mode // if true, Karma captures browsers, runs the tests and exits singleRun: false, // Concurrency level // how many browser should be started simultaneous concurrency: Infinity, plugins : [ 'karma-requirejs', 'karma-jasmine', 'karma-chrome-launcher', 'karma-firefox-launcher', 'karma-browserify' ], frameworks: ['jasmine', 'browserify'] }) }
Is there something obvious I'm doing wrong here? I'm hoping there is, it looks like I've halfway implemented a couple solutions here without exactly knowing what is going on. Thanks!
I'm getting the error on my app.js file on the first line with 'require'