URL Redirect Based on Screen Width
Solution 1
I'm answering my own question as I have recently found a more satisfying solution then the one provided by @Finduilas.
This solution not only redirects you in normal circumstances, it will also redirect you back and forth as you resize your window.
Even better! It will redirect you back and forth in mobile devices as you switch from landscape to portrait and vice-versa.
<script type="text/javascript">
$(window).on('load resize',function(){
if($(window).width() < 950){
window.location = "https://www.google.com"
}
});
</script>
Solution 2
If you have to use Javascript, try the following:
<script type="text/javascript">
function redirect() {
if (screen.width <= 950) {
window.location = "https://www.google.com/";
}
and in your body add :
<body onload="setTimeout('redirect()', 300)">
This will redirect the user after 300 ms when the page is loaded, this way you are making sure that the width
of the screen is available.
Solution 3
You can better use JQUERY for this...
// Returns width of browser viewport
$( window ).width();
See: http://api.jquery.com/width/
Example:
<!DOCTYPE html>
<html>
<head>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<script>
$(document).ready(function(){
if($(window).width() <= 950) {
window.location = "https://www.google.com/";
}
});
</script>
</head>
<body>
<p>Website</p>
</body>
</html>
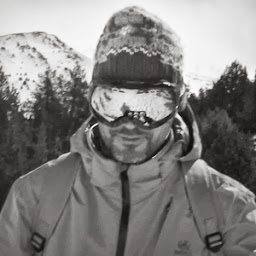
David Martins
Updated on January 13, 2022Comments
-
David Martins over 2 years
What I'm looking for is quite simply, a way to automatically redirect the user to a different URL when the screen or window width is less then 950px.
I don't want to use "refresh" as it's not recommended, and I don't do want to use "User Agent" either as it seams to me less reliable in the long term and I don't want to be concerned with updating this.
This is the Script I see suggested everywhere for this purpose but for some reason it doesn't do anything:
<script type="text/javascript"> <!-- if (screen.width <= 950) { window.location = "https://www.google.com/"; } //--> </script>
I've also tried it with all this variants with no success:
window.location document.location window.location.href document.location.href
I've also tried placing it as the first thing after Head tag and even before Doctype. Nothing happens...