Use Variable Which is in main method in Another Method
13,576
Solution 1
import java.util.Scanner;
public class Alpha
{
public static void main(String args[])
{
Scanner input = new Scanner(System.in);
int n;
System.out.println("Enter no. of stars");
n = input.nextInt();
Loop(n); //calls Loop function and passes parameter n
}
public static void Loop(int n) //this function now expects a number n
{
for (int counter = 1; counter <= n; counter++)
{
System.out.println("*");
}
}
}
Solution 2
Pass it as a paramteer
import java.util.Scanner;
public class Alpha
{
public static void main(String args[])
{
Scanner input = new Scanner(System.in);
int n;
System.out.println("Enter no. of stars");
n = input.nextInt();
loop(n); // added this
}
public static void loop (int n) // changed here
{
for (int counter = 1; counter <= n; counter++)
{
System.out.println("*");
}
}
}
Solution 3
simply pass it as parameter:
public static void main(String args[])
{
Scanner input = new Scanner(System.in);
int n;
System.out.println("Enter no. of stars");
n = input.nextInt();
Loop(n);
}
public static void Loop (int count)
{
for (int counter = 1; counter <= count; counter++)
{
System.out.println("*");
}
}
Solution 4
I think you should use it as a instance variable and for better understanding name your class like StarClass
it can provide better understanding. Good programming practice.
But you should avoid unneccesserily making instance variable without any logic behind it.
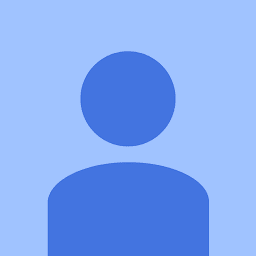
Author by
ichigo gyuunyuu
Updated on June 09, 2022Comments
-
ichigo gyuunyuu about 2 years
I'm trying to create a simple program to output the number of stars entered by user. I'm trying to learn how to use more than one method to do this Here's my code
import java.util.Scanner; public class Alpha { public static void main(String args[]) { Scanner input = new Scanner(System.in); int n; System.out.println("Enter no. of stars"); n = input.nextInt(); } public static void Loop () { for (int counter = 1; counter <= n; counter++) { System.out.println("*"); } } }
The problem I'm facing is that in the Loop method, I am unable to use the variable n Is there a way to use a variable which is in the main method, in another one? Ty
-Pingu