Using Malloc Hooks
Solution 1
Did you look here? http://www.gnu.org/software/libtool/manual/libc/Hooks-for-Malloc.html
Solution 2
According to http://www.gnu.org/software/libtool/manual/libc/Hooks-for-Malloc.html, here's how to do this with the GCC libraries.
/* Prototypes for __malloc_hook, __free_hook */
#include <malloc.h>
/* Prototypes for our hooks. */
static void my_init_hook (void);
static void *my_malloc_hook (size_t, const void *);
static void my_free_hook (void*, const void *);
/* Override initializing hook from the C library. */
void (*__malloc_initialize_hook) (void) = my_init_hook;
static void
my_init_hook (void)
{
old_malloc_hook = __malloc_hook;
old_free_hook = __free_hook;
__malloc_hook = my_malloc_hook;
__free_hook = my_free_hook;
}
static void *
my_malloc_hook (size_t size, const void *caller)
{
void *result;
/* Restore all old hooks */
__malloc_hook = old_malloc_hook;
__free_hook = old_free_hook;
/* Call recursively */
result = malloc (size);
/* Save underlying hooks */
old_malloc_hook = __malloc_hook;
old_free_hook = __free_hook;
/* printf might call malloc, so protect it too. */
printf ("malloc (%u) returns %p\n", (unsigned int) size, result);
/* Restore our own hooks */
__malloc_hook = my_malloc_hook;
__free_hook = my_free_hook;
return result;
}
static void
my_free_hook (void *ptr, const void *caller)
{
/* Restore all old hooks */
__malloc_hook = old_malloc_hook;
__free_hook = old_free_hook;
/* Call recursively */
free (ptr);
/* Save underlying hooks */
old_malloc_hook = __malloc_hook;
old_free_hook = __free_hook;
/* printf might call free, so protect it too. */
printf ("freed pointer %p\n", ptr);
/* Restore our own hooks */
__malloc_hook = my_malloc_hook;
__free_hook = my_free_hook;
}
main ()
{
...
}
Solution 3
If your function calls sbrk directly you can simply call it malloc and make sure it gets linked in before the library's malloc. This works on all Unix type OSes. For windows see Is there a way to redefine malloc at link time on Windows?
If your function is a wrapper around the library's malloc, the #define suggestion by Alex will work.
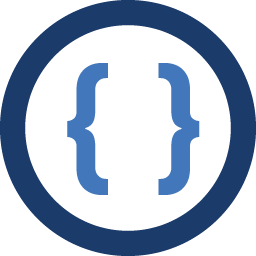
Admin
Updated on August 16, 2022Comments
-
Admin almost 2 years
I am trying to use a malloc hook to create a custom function my_malloc(). In my main program when I call malloc() I want it to call my_malloc() can someone please give me an example on how to do this in C
-
Admin over 15 yearsso if i create a include file my_malloc.c and include it into my main program and just do that it will call my_malloc instead of malloc?
-
Graeme Perrow over 15 yearsThis will only work for calls directly to malloc() in that file. Any malloc calls in functions in other files (or in a library that you don't control) will not be affected. If you do this, however, you should probably have a my_realloc as well.
-
philant over 15 years#undefine is not a valid C preprocesser directive, it's #undef
-
Heath Hunnicutt over 14 yearsFor good reason you could prefer #define malloc(s) my_malloc(s,...), such as #define malloc(s) my_malloc(s, FILE, LINE) and similar. Also using the macro with arguments allows one to call (malloc)(s) in those cases where one does desire the underlying symbol.
-
Fei Xue almost 12 yearswhat does ` old_malloc_hook = __malloc_hook;` mean? I am confused. We set
__malloc_hook
toold_malloc_hook
before, why we save__malloc_hook
toold_malloc_hook
again? My question is here stackoverflow.com/questions/11356958/how-to-use-malloc-hook/… -
Randy Stegbauer almost 12 yearsDavid Schwartz mentioned in his answer to stackoverflow.com/questions/11356958/how-to-use-malloc-hook that saving the original _malloc_hook is important so that it can be restored just before the original malloc() is called. That's the line just below the comment /* Restore all old hooks */. I'm guessing that in this specific case, it's unnecessary, since the original malloc hook is null, but to be safe it should be done. It must be save in all three of the "my" functions. In my_malloc and my_free, it must be done just before calling the system's malloc and free functions.