Using Sweet alert with Typescript class
Solution 1
Typescript
I got it to work with typescript the following way. In the top of your .ts
file use:
import * as swal from 'sweetalert';
Then use the function like this
swal({
title: "Are you sure?",
text: "You will not be able to undo this action",
type: "warning",
showCancelButton: true,
confirmButtonColor: "#DD6B55",
confirmButtonText: "Yes, delete it!",
closeOnConfirm: true
},
confirmed => {
if(confirmed) {
// Do what ever when the user click on the 'Yes, delete it' button
}
});
You can find more examples in the docs.
Installation via npm and typings
I installed the sweetalert
package the via npm
npm install sweetalert --save
and the typings
// the old way
typings install dt~sweetalert --save --global
// new way
npm install --save @types/sweetalert
After this, make sure you have included the js and the css file in your index.html.
If you get the following error
swal(..) is not a function
then you have not included the js file properly.
For aurelia CLI users
You can add the following to your aurelia.json
.
{
"name": "sweetalert",
"path": "../node_modules/sweetalert",
"main": "dist/sweetalert.min",
"resources": [
"dist/sweetalert.css"
]
},
and in app.html use
<require from="sweetalert/dist/sweetalert.css"></require>
Solution 2
I know it's an old question but the problem with SweetAlert2 and Angular still remain (I use Angular 9 and Sweetalert2 version 8.19.0)... After some hours of headheaches I found the final solution to this problem without any hack.
We can't use the function swal({...})
but we can use the second overload of Swal.fire({...})
method, casting the parameters to SweetAlertOptions...
My code looks like this...
import Swal, {SweetAlertOptions} from 'sweetalert2';
Swal.fire({
title: 'Are you sure?',
text: 'You won\'t be able to revert this!',
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
} as SweetAlertOptions).then((result) => {
if (result.value) {
//Do something here
}
});
Related videos on Youtube
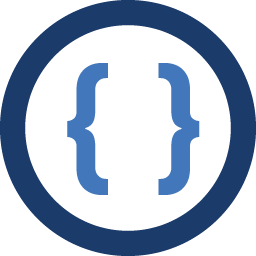
Admin
Updated on July 01, 2022Comments
-
Admin about 2 years
I have a method inside a typescript class that just looks like this
var confirmation = confirm("Run Agent Job?"); if (confirmation) { console.log('yes'); } else { console.log('no'); }
I'm trying to convert this to use Sweet Alert, so inside the method I just put this. But Typescript doesn't recognize this It throws a
Cannot find name swal
swal("hello");
I have imported sweet alert as follows
<link href="~/Content/css/sweetalert.css" rel="stylesheet" /> <script src="~/Scripts/sweetalert.min.js"></script>
What am I doing wrong? If I try to use
swal()
in just a plain*.js
file, it'll work fine. It's only when it's in a*.ts
file. -
Aman B over 5 yearsIt's better to paste the code as text instead of an image. Images could be lost if some is coming here after years
-
mliebelt over 2 yearsSaved my day, thx!