Using the SQL Command object, how can you check to see if the result set is empty?
Solution 1
Check out the definition of ExecuteScalar. It returns an Object, which will have a null reference if the result set is empty.
The reason you are seeing zero is that Convert.ToInt32
returns a zero when given null
. You need to check the return value from ExecuteScalar
before you convert it to an int
.
Solution 2
DbCommand.ExecuteScalar() returns the first column of the first row, or null if the result is empty. Your problem is caused by Convert.ToInt32() because it returns 0 for null.
You have to check the value returned by ExecuteScalar() for null and only call Convert.ToInt32() if it is not null.
Object result = command.ExecuteScalar();
if (result != null)
{
Int32 value = Convert.ToInt32(result);
}
else
{
// Handle the empty result set case
}
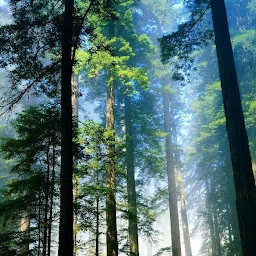
ahmed galal
Updated on June 14, 2022Comments
-
ahmed galal almost 2 years
I using quarkus 8.3.Final , gradle Native build always get stucked at
Building native image source jar: /home/runner/work/qu-queue-service/qu-queue-service/build/qu-queue-service-1.0.0-SNAPSHOT-native-image-source-jar/qu-queue-service-1.0.0-SNAPSHOT-runner.jar Building native image from /home/runner/work/qu-queue-service/qu-queue-service/build/qu-queue-service-1.0.0-SNAPSHOT-native-image-source-jar/qu-queue-service-1.0.0-SNAPSHOT-runner.jar Using docker to run the native image builder Checking image status quay.io/quarkus/ubi-quarkus-mandrel:22.0.0.2-Final-java17 22.0.0.2-Final-java17: Pulling from quarkus/ubi-quarkus-mandrel 54e56e6f8572: Pulling fs layer 4f8ddd7f5a75: Pulling fs layer 20939a5b3d59: Pulling fs layer 4f8ddd7f5a75: Verifying Checksum 4f8ddd7f5a75: Download complete 54e56e6f8572: Verifying Checksum 54e56e6f8572: Download complete 54e56e6f8572: Pull complete 20939a5b3d59: Verifying Checksum 20939a5b3d59: Download complete 4f8ddd7f5a75: Pull complete 20939a5b3d59: Pull complete Digest: sha256:7751b408ac408d6f91a95c864a2b8d85129987c8d5c1fc5356e9940c8e330837 Status: Downloaded newer image for quay.io/quarkus/ubi-quarkus-mandrel:22.0.0.2-Final-java17 quay.io/quarkus/ubi-quarkus-mandrel:22.0.0.2-Final-java17 Running Quarkus native-image plugin on native-image 22.0.0.2-Final Mandrel Distribution (Java Version 17.0.2+8) docker run --env LANG=C --rm --user 1001:121 -v /home/runner/work/qu-queue-service/qu-queue-service/build/qu-queue-service-1.0.0-SNAPSHOT-native-image-source-jar:/project:z --name build-native-clyaI quay.io/quarkus/ubi-quarkus-mandrel:22.0.0.2-Final-java17 -J-Dsun.nio.ch.maxUpdateArraySize=100 -J-Djava.util.logging.manager=org.jboss.logmanager.LogManager -J-DCoordinatorEnvironmentBean.transactionStatusManagerEnable=false -J-Dcom.sun.xml.bind.v2.bytecode.ClassTailor.noOptimize=true -J-Dvertx.logger-delegate-factory-class-name=io.quarkus.vertx.core.runtime.VertxLogDelegateFactory -J-Dvertx.disableDnsResolver=true -J-Dio.netty.leakDetection.level=DISABLED -J-Dio.netty.allocator.maxOrder=3 -J-Duser.language=en -J-Dfile.encoding=UTF-8 -H:-ParseOnce -J--add-exports=java.security.jgss/sun.security.krb5=ALL-UNNAMED -J--add-opens=java.base/java.text=ALL-UNNAMED -H:InitialCollectionPolicy=com.oracle.svm.core.genscavenge.CollectionPolicy\$BySpaceAndTime -H:+JNI -H:+AllowFoldMethods -J-Djava.awt.headless=true -H:FallbackThreshold=0 -H:+ReportExceptionStackTraces -J-Xmx3G -H:-AddAllCharsets -H:EnableURLProtocols=http,https -H:-UseServiceLoaderFeature -H:+StackTrace qu-queue-service-1.0.0-SNAPSHOT-runner -jar qu-queue-service-1.0.0-SNAPSHOT-runner.jar ======================================================================================================================== GraalVM Native Image: Generating 'qu-queue-service-1.0.0-SNAPSHOT-runner'... ======================================================================================================================== [1/7] Initializing... (13.1s @ 0.22GB) Version info: 'GraalVM 22.0.0.2-Final Java 17 Mandrel Distribution' 8 user-provided feature(s) - io.quarkus.caffeine.runtime.graal.CacheConstructorsAutofeature - io.quarkus.hibernate.orm.runtime.graal.DisableLoggingAutoFeature - io.quarkus.jdbc.postgresql.runtime.graal.SQLXMLFeature - io.quarkus.runner.AutoFeature - io.quarkus.runtime.graal.DisableLoggingAutoFeature - io.quarkus.runtime.graal.ResourcesFeature - org.hibernate.graalvm.internal.GraalVMStaticAutofeature - org.hibernate.graalvm.internal.QueryParsingSupport [2/7] Performing analysis... [**********] (191.2s @ 2.47GB) 26,471 (96.76%) of 27,356 classes reachable 45,531 (68.17%) of 66,791 fields reachable 143,995 (80.30%) of 179,318 methods reachable 1,537 classes, 1,795 fields, and 10,535 methods registered for reflection 65 classes, 77 fields, and 55 methods registered for JNI access [3/7] Building universe... (7.2s @ 2.60GB) [4/7] Parsing methods... [******] (36.8s @ 2.34GB)
The last build took 6h on github CI, on my local machine it also stop there while grinding the CPU
Is there any leads I can follow?