VBScript and loadXML: Invalid at the top level of the document. How to fix it?
Solution 1
xmlDoc.loadXML()
can load an XML string. It cannot retrieve a URL.
Use an XMLHTTPRequest object if you need to make an HTTP request.
Function LoadXml(xmlurl)
Dim xmlhttp
Set xmlhttp = CreateObject("MSXML2.XMLHTTP")
xmlhttp.Open "GET", xmlurl, false
' switch to manual error handling
On Error Resume Next
xmlhttp.Send
If err.number <> 0 Then
WScript.Echo xmlhttp.parseError.Reason
Err.Clear
End If
' switch back to automatic error handling
On Error Goto 0
Set LoadXml = xmlhttp.ResponseXml
End Function
Use like
Set doc = LoadXml("http://your.url/here")
Solution 2
Three addition remarks:
(1) As .parseError.reason tends to be cryptic, it pays to include its .srcTxt property (and the parameter to .loadXml):
Dim xmlurl : xmlurl = "song.xml"
Dim xmlDoc : Set xmlDoc = CreateObject("Microsoft.XMLDOM")
xmlDoc.async = False
xmlDoc.loadXML xmlurl
If 0 <> xmlDoc.parseError.errorcode Then
WScript.Echo xmlDoc.parseError.reason, "Src:", xmlDoc.parseError.srcText
Else
WScript.Echo "surprise, surprise"
End if
output:
Invalid at the top level of the document.
Src: song.xml
Of course, writing a Function/Sub that takes all properties of .parseError into account and using that always, would be even better.
(2) To load a file or URL, use .load:
Dim xmlDoc : Set xmlDoc = CreateObject("Microsoft.XMLDOM")
Dim xmlurl
For Each xmlurl In Array("song.xml", "http://gent/~eh/song.xml", "zilch")
xmlDoc.async = False
if xmlDoc.load(xmlurl) Then
With xmlDoc.documentElement.firstChild
WScript.Echo xmlurl _
, .tagName _
, .firstChild.tagName _
, .firstChild.text
End With
Else
WScript.Echo xmlurl, xmlDoc.parseError.reason, "Src:", xmlDoc.parseError.srcText
End if
Next
output:
song.xml nowplaying-info property CKOI-ÄÖÜ
http://gent/~eh/song.xml nowplaying-info property CKOI-ÄÖÜ
zilch The system cannot locate the object specified.
Src:
(3) Using the DOM avoids all encoding problems (that's why I put some german umlauts into 'your' file - which even made it to the DOS-Box output) and makes RegExps (even Perl's) a second best choice.
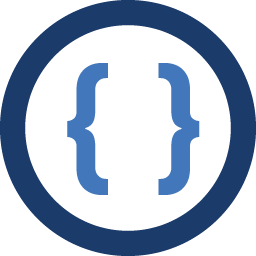
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
This is my fort post on stackoverflow. I have searched many similiar Q&A's on this site but my conditions seem a bit different. here is my vbscript code:
------------ code snippet ---------------
xmlurl = "songs.xml" set xmlDoc = CreateObject("Microsoft.XMLDOM") xmlDoc.async = False xmlDoc.loadXML(xmlurl) if xmlDoc.parseError.errorcode<>0 then 'error handling code msgbox("error! " & xmlDoc.parseError.reason) end if
------------ end code snippet ---------------
XML:
<?xml version="1.0" encoding="UTF-8"?> <nowplaying-info-list> <nowplaying-info mountName="CKOIFMAAC" timestamp="1339771946" type="track"> <property name="track_artist_name"><![CDATA[CKOI]]></property> <property name="cue_title"><![CDATA[HITMIX]]></property> </nowplaying-info> <nowplaying-info mountName="CKOIFMAAC" timestamp="1339771364" type="track"> <property name="track_artist_name"><![CDATA[AMYLIE]]></property> <property name="cue_title"><![CDATA[LES FILLES]]></property> </nowplaying-info> <nowplaying-info mountName="CKOIFMAAC" timestamp="1339771149" type="track"> <property name="track_artist_name"><![CDATA[MIA MARTINA]]></property> <property name="cue_title"><![CDATA[TOI ET MOI]]></property> </nowplaying-info> </nowplaying-info-list>
I also tried removing the first line in case maybe UTF-8 was not compatible with windows (saw some posts about this), but I still got the same error. I also tried unix2dos and vice versa in case there were carriage return issues (hidden characters embedded in the xml). I just can't seem to figure out what's wrong. It's such a simole XML file. I could parse it in a few minutes using perl regex but I need to run this script on windows so using vbscript. I use the same technique to parse XML from other sources without any issues. I cannot modify the XML unfortunately, it is from an external source. I have this exact same error on both my Windows Vista home edition and Windows Server 2008. I am running the vbscript from the command line for testing so far (ie not in ASP).
Thanks in advance,
Sam