Vertical timetable flutter
So after some more research, I arrived at a solution for this problem.
The vertical lists
In order to construct the vertical lists, I used the following code. This constructs one timetable for an asset.
const totalWidth = 2400.0; // Constant for all calculations. 24 hours in a day! 100 pixels per hour.
Widget _createTimetable(SomeData data) {
return Column(
children: [
Container(
padding: EdgeInsets.all(2),
decoration: BoxDecoration(
color: Colors.grey,
),
width: double.infinity,
child: Text("${data.name}", style: TextStyle(color: Colors.white)),
),
Container(
height: 40, // Fixed height
child: ListView(
scrollDirection: Axis.horizontal,
controller: _controllers[data.name], // Controller, see next section
physics: ClampingScrollPhysics(),
shrinkWrap: true,
children: [
Container(
width: totalWidth,
color: Colors.white,
child: Stack(
children: [Positioned(left: timeOfsset), bottom: 0, top: 0, child: <Some Container>]
)),
]
)
)
],
);
This approach uses a container that has a static width. This container is put into the ListView to make it scrollable. The container in turn houses a Stack widget. This widget allows the placement of widgets using the Positioned widget. The advantage of this is that they do not interfere with each other. This makes the positioning rather easy (after doing some math!).
Linking the lists
In order to link the ListController objects for each list, I used the linked_scroll_controller
package. This allows you to generate the controllers and link them together. Make sure to dispose of them probably when the widget is disposed of.
In order to get the timestamp at the top, I used an additional vertical view that uses the same method. It's linked together with the other controllers.
Conclusion
This solution is not ideal yet I think. However, it works! For the calculations of the offSet and width of the 'reservations, some helper functions were made. They take the total width into account.
That's all really! Hope this will help some other people in the future. If you have any improvements feel free to comment or edit.
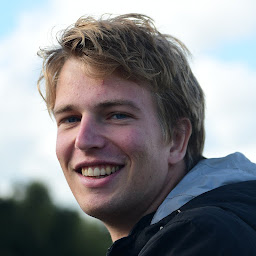
Marten
Updated on January 03, 2023Comments
-
Marten over 1 year
I am quite new to Flutter and I am enjoying the framework very much. However, I have been getting stuck on the following:
The problem: Users need to be able to make reservations for certain sports assets. I decided to go with the following approach regarding the UI.
Concept layout
The layout features a row with the name of the asset. After that a vertical vertical scrolling row (all rows scroll at the same time such that it's easy to look up a free spot). These vertical scrolling bars contain the reservations, allowing you to scroll through all hours of the day.
I tried finding an existing layout that would help me here, sadly with no luck. Having tried some methods of my own (horizontal listview with vertical listviews) I did not manage to solve this problem.
The questions I have now are the following:
- Did I miss a framework that already solves this issue?
- How would one go about spacing out the items in the vertical listview (regarding time 'slots')? Using Containers with margins seems a bit harsh.
- Is it possible to sync all vertical listviews with each other?
Any hints or answers are much appreciated!