Flutter: How to call images from API
What you are getting from the API is the already pre rendered html content. The code you posted in the first screenshot is HTML code, you can run it inside any .html
file. What you need is to strip the images from it.
Would suggest first thing is to try to get another wordpress API if possible, to return the images relating to a specific post.
If you cannot do that, attempt the following:
Parse the entire response as a string.
Use string interpolation to perform a series of
split()
methods.First, split by the pattern which is your base url, i.e
http://thewriting.../uploads/
. The above will give you aList<String>
, each element will start with what is after/uploads/
and ends withsomeImageUrl.jpg somenumber.w
.On this list, perform a
forEach()
loop, where you iterate over every element, and then use.split()
and use the pattern".jpg"
. It will give you a list of two string, the first element is the url you want, the second element is rubish you don't need.Inside the same for each, after you do the split, you take the first
[0]
entry.after that, you concatenate your baseUrl, with the image path with
.jpg
. And you get you array of paths.
final String _baseUrl = 'http://thewriting..../uploads/'; //write the entire url until uploads.
List<String> _list = yourHtmlResponse.split(_baseUrl);
_list.forEach((element) {
element =_baseUrl + element.split('.jpg').first + '.jpg';
print(element);//just to check how the url is looking so far.
});
The above should get you a List<String>
which has all the images in the response.
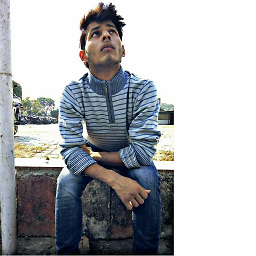
Bhaskar2510
Updated on January 04, 2023Comments
-
Bhaskar2510 20 minutes
I have a WordPress blog and I'm trying to create an app for that blog. While using an API for the blog I'm unable to find the right set of code to fetch images that are in between the blog I have fetched the featured image of the blog but the problem is to fetch the images between paragraph text. For now, I'm getting such texts instead of the images. Screenshots attached.
Secondly, While fetching the text I'm getting the tags along with the text so how can I remove these tags. Screenshot attached.
Here is my code which I'm using to fetch all of these:
import 'package:flutter/material.dart'; import 'package:thewritingparadigm/screens/post_details.dart'; import 'package:thewritingparadigm/service/post_data.dart'; class LatestPost extends StatefulWidget { const LatestPost({Key? key}) : super(key: key); @override State<LatestPost> createState() => _LatestPostState(); } class _LatestPostState extends State<LatestPost> { Post postService = Post(); @override Widget build(BuildContext context) { return FutureBuilder<List>( future: postService.getAllPost(), builder: (context, snapshot) { if (snapshot.hasData) { if (snapshot.data!.isEmpty) { return const Center(child: Text("No Post Available")); } return ListView.builder( shrinkWrap: true, itemCount: snapshot.data?.length, itemBuilder: (context, i) { return Card( child: ListTile( title: Column( children: [ const SizedBox( height: 20.0, ), SizedBox( width: double.infinity, height: 250.0, child: Image.network(snapshot.data![i]["_embedded"] !["wp:featuredmedia"][0]["source_url"]), ), const SizedBox( height: 15.0, ), Text( snapshot.data![i]['title']['rendered'], style: const TextStyle( fontSize: 25.0, fontWeight: FontWeight.bold, ), ), ], ), subtitle: Container( padding: const EdgeInsets.only( left: 5.0, right: 5.0, bottom: 25.0), child: Text( snapshot.data![i]['content']['rendered'] .toString() .replaceAll("<p>", "") .replaceAll("</p>", ""), maxLines: 4, overflow: TextOverflow.ellipsis, style: const TextStyle(fontSize: 16.0), ), ), onTap: () { Navigator.push( context, MaterialPageRoute( builder: (context) => PostDetails(data: snapshot.data?[i]), ), ); }, ), ); }); } else if (snapshot.hasError) { return Center( child: Text(snapshot.error.toString()), ); } else { return const Center( child: CircularProgressIndicator(), ); } }, ); } }
-
Ravindra S. Patil 7 monthsI don't understand your problem can you explain in brief?
-
Bhaskar2510 7 monthsHey! I've updated my question hope I have conveyed my problem so that you can help me out.
-
Shawon 7 monthsI think you fetch your API response as an HTML block. please receive your API response as a JSON object.
-
Bhaskar2510 7 monthsActually, it's my first time using API so if you could give me some examples it would be great if it would be from my code itself.
-
Pyzard 7 monthsAs Huthaifa said, what you're getting is the HTML content of the site. If I were in your situation, I would make an API using Python
Flask
that scrapes the website withBeautifulSoup4
and returns just the required information. -
anggadaz 7 monthsuse parser to delete html tag. widget only need image
-
Kaushik Chandru 7 monthsCan you add your response here too please?
-
-
Bhaskar2510 7 monthsThere is no library there.
-
keyur 7 monthsSorry, Please check again