Flutter: use 2 classes when using StateMixin in getx controller
174
Yes you can! And as you guessed it, by inheritance.
At first create a generic State class containing the types you want:
class MyState<T1, T2> { // you can use more like T3, T4...
T1? state1;
T2? state2;
MyState({this.state1, this.state2});
}
Then create a base controller of your own:
abstract class BaseController<T1, T2> extends GetxController with StateMixin<MyState<T1, T2>> {
}
Then extend this base controller:
class HomeController extends BaseController<int, String> {
@override
void onInit() {
super.onInit();
change(null, status: RxStatus.loading());
Future.delayed(Duration(seconds: 3), () {
change(MyState(state1:1,state2: "state2"), status:RxStatus.success());
});
}
}
Then on your widget:
controller.obx(
(state) => Text("${state?.state1} - ${state?.state2}"),
)
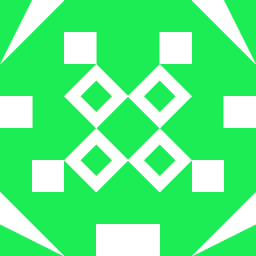
Author by
Cyrus the Great
Updated on January 04, 2023Comments
-
Cyrus the Great 13 minutes
I am using getx as a statemanagement on my project. I created a controller and I am using StateMixin on my controller.
class HomeController extends GetxController with StateMixin<MyModel, List<MyCompleteModel>> {
As you can see I want to use 2 classes by
StateMixin
. But it's constructor just accepts one class. Except create a new class that contains both of these classes, Is there any way to fix this problem?