Video player breaks down in flutter 2.0 (null safety)
MEDIA_ERR_SRC_NOT_SUPPORTED
is just a consequence of another error net::ERR_CERT_COMMON_NAME_INVALID
(you can see it in the browser console). Because not all SSL certificates cover both the WWW and non-WWW versions of a website by default (as in case with sample-videos.com), you have to remove www
from url:
_controller = VideoPlayerController.network(
'https://sample-videos.com/video123/mp4/720/big_buck_bunny_720p_20mb.mp4')
..initialize().then((_) {
// Ensure the first frame is shown after the video is initialized, even before the play button has been pressed.
setState(() {});
});
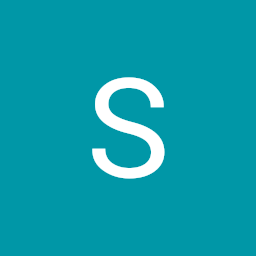
Siddharth Agrawal
Updated on November 23, 2022Comments
-
Siddharth Agrawal over 1 year
I want to show a video in my flutter app and like always, I am using the video_player plugin. But for some reason, now that I have migrated to null safety, the video player gives me this error
Error: PlatformException(MEDIA_ERR_SRC_NOT_SUPPORTED, MEDIA_ELEMENT_ERROR: Format error, The video has been found to be unsuitable (missing or in a format not supported by your browser)., null) at Object.createErrorWithStack (http://localhost:59815/dart_sdk.js:5050:12) at Object._rethrow (http://localhost:59815/dart_sdk.js:37641:16) at async._AsyncCallbackEntry.new.callback (http://localhost:59815/dart_sdk.js:37637:13) at Object._microtaskLoop (http://localhost:59815/dart_sdk.js:37497:13) at _startMicrotaskLoop (http://localhost:59815/dart_sdk.js:37503:13) at http://localhost:59815/dart_sdk.js:33274:9
I am running my code on flutter web, and am using the exact copy paste of the example code, just migrated to null safety. This is my code
import 'package:video_player/video_player.dart'; import 'package:flutter/material.dart'; void main() => runApp(ChapterVideoPlayer()); class ChapterVideoPlayer extends StatefulWidget { @override _ChapterVideoPlayerState createState() => _ChapterVideoPlayerState(); } class _ChapterVideoPlayerState extends State<ChapterVideoPlayer> { VideoPlayerController? _controller; @override void initState() { super.initState(); _controller = VideoPlayerController.network( 'https://www.sample-videos.com/video123/mp4/720/big_buck_bunny_720p_20mb.mp4') ..initialize().then((_) { // Ensure the first frame is shown after the video is initialized, even before the play button has been pressed. setState(() {}); }); } @override Widget build(BuildContext context) { return MaterialApp( title: 'Video Demo', home: Scaffold( body: Center( child: _controller!.value.isInitialized ? AspectRatio( aspectRatio: _controller!.value.aspectRatio, child: VideoPlayer(_controller!), ) : Container(), ), floatingActionButton: FloatingActionButton( onPressed: () { setState(() { _controller!.value.isPlaying ? _controller!.pause() : _controller!.play(); }); }, child: Icon( _controller!.value.isPlaying ? Icons.pause : Icons.play_arrow, ), ), ), ); } @override void dispose() { super.dispose(); _controller!.dispose(); } }
Am I doing it wrong? Or is there a problem with the plugin. Is there any replacement I can use for the meanwhile? Any help would be very appreciated. Iam using version
^2.1.1