Webpack 4. Compile scss to separate css file
Solution 1
Other answers gave me just headache as they were not working. I did a lot of googling and I realized you can compile scss
into separate css
file without using any additional plugins
webpack.config.js
const path = require('path');
module.exports = {
entry: [
__dirname + '/src/js/app.js',
__dirname + '/src/scss/app.scss'
],
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'js/app.min.js',
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: [],
}, {
test: /\.scss$/,
exclude: /node_modules/,
use: [
{
loader: 'file-loader',
options: { outputPath: 'css/', name: '[name].min.css'}
},
'sass-loader'
]
}
]
}
};
package.json
{
"name": "...",
"version": "1.0.0",
"description": "...",
"private": true,
"dependencies": {},
"devDependencies": {
"file-loader": "^5.0.2",
"node-sass": "^4.13.1",
"sass-loader": "^8.0.2",
"webpack": "^4.41.5",
"webpack-cli": "^3.3.10"
},
"scripts": {
"build": "webpack --config webpack.config.js --mode='production'"
},
"keywords": [],
"author": "...",
"license": "ISC"
}
Dependencies:
npm install --save-dev webpack webpack-cli file-loader node-sass sass-loader
How to run JS and SCSS compilation
npm run build
Solution 2
You can use the MiniCssExtractPlugin. This will extract your css into a separate file.
There are a few parts of your webpack.config.js file you'll need to add to, or change.
You'll need to require the plugin at the top of the file:
const MiniCssExtractPlugin = require("mini-css-extract-plugin");
A plugins property is also required in the modules object:
plugins: [
new MiniCssExtractPlugin({
filename: "[name].css",
chunkFilename: "[id].css"
})
]
And you'll need to change your scss rule. Note the test is slightly different to include .scss files (probably best to name you scss files .scss) and the addition of the sass-loader which you'll need to install with npm. The loaders in the 'use' array operate in reverse order, so sass-loaded goes first, converting scss to css, then the css-loader and then extract plugin extracts the css out again to a separate file:
{
test: /\.s?css$/,
use: [
MiniCssExtractPlugin.loader,
"css-loader",
"sass-loader"
]
}
So I think your config file will change to this:
const MiniCssExtractPlugin = require("mini-css-extract-plugin");
var path = require("path");
module.exports = {
entry: "./js/main.js",
output: {
path: path.resolve(__dirname, "dist"),
filename: "bundle.js",
publicPath: "/dist"
},
watch:true,
module: {
rules: [
{
test: /\.js$/,
use: {
loader: "babel-loader",
options: { presets: ["es2015"] }
}
},
{
test: /\.s?css$/,
use: [
MiniCssExtractPlugin.loader,
"css-loader",
"sass-loader"
]
}
]
},
plugins: [
new MiniCssExtractPlugin({
filename: "[name].css",
chunkFilename: "[id].css"
})
]
}
Hope that helps.
Solution 3
to update @Fusion 's anwser:
from https://github.com/webpack-contrib/file-loader:
DEPRECATED for v5: please consider migrating to asset modules.
the official docs (https://webpack.js.org/guides/asset-modules/) mention that clearly: as of webpack v5, raw-loader
, url-loader
, file-loader
loaders are now depricated and replaced with Asset Modules, and new 4 module types: asset/resource
(which replace file-loader
), asset/inline
, asset/source
, asset
are introduced.
so, the new way to compile scss to separate css file:
const path = require('path');
module.exports = {
entry: [
__dirname + '/src/js/app.js',
__dirname + '/src/scss/app.scss'
],
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'js/app.min.js',
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: [],
}, {
test: /\.scss$/,
exclude: /node_modules/,
type: 'asset/resource',
generator: {
filename: 'css/[name].min.css'
},
use: [
{
loader: 'file-loader',
options: { outputPath: 'css/', name: '[name].min.css'}
},
'sass-loader'
]
}
]
}
};
the big advantage is that Asset Modules is a webpack built-in and you won't even need to install any 3rd module.
refer to:
- https://webpack.js.org/guides/asset-modules/#resource-assets
- https://webpack.js.org/guides/asset-modules/#custom-output-filename
Update:
you may realise that webpack generates an extra/unwanted/unexpected js file for non-js files (css, scss ...) despite the methode you use file-loader
or asset
modules, in this case, https://www.npmjs.com/package/webpack-fix-style-only-entries comes handy to solve this limitation.
const path = require('path');
const FixStyleOnlyEntriesPlugin = require("webpack-fix-style-only-entries");
module.exports = {
entry: [
__dirname + '/src/js/app.js',
__dirname + '/src/scss/app.scss'
],
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'js/app.min.js',
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: [],
}, {
test: /\.scss$/,
exclude: /node_modules/,
type: 'asset/resource',
generator: {
filename: 'css/[name].min.css'
},
use: [
'sass-loader'
]
}
]
},
plugins: [
new FixStyleOnlyEntriesPlugin({ extensions:['scss'] }),
],
};
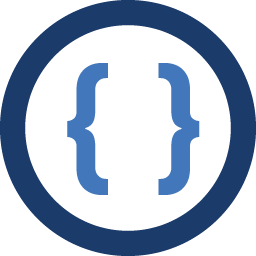
Admin
Updated on February 02, 2022Comments
-
Admin about 2 years
Im trying to compile scss into a separate css file with no luck. As it is now the css gets into the bundle.js together with all js code. How can i separate my css into its own file?
This is how my config looks:
var path = require("path"); module.exports = { entry: "./js/main.js", output: { path: path.resolve(__dirname, "dist"), filename: "bundle.js", publicPath: "/dist" }, watch:true, module: { rules: [ { test: /\.js$/, use: { loader: "babel-loader", options: { presets: ["es2015"] } } }, { test: /\.scss$/, use: [ { loader: "style-loader" }, { loader: "css-loader" }, { loader: "sass-loader" } ] } ] } };
-
Admin almost 6 yearsUnderstood. When you have installed the npm module, how do you get it to actually work? I mean, how would you change the webpack-file?
-
Admin almost 6 yearsThanks! it helped me a bit, but it does not create any css file. I have updated the code in my post.
-
Bryan almost 6 years@fortyfiveknots hmm, ok. I will look later, but until this evening. Do you get any errors?
-
Bryan almost 6 years@fortyfiveknots I have update my answer. I never had the loaders quite right.
-
Ari about 5 yearsIt can be done without mini-css-plugin. please see the answer of my question here stackoverflow.com/questions/55035376/…
-
Joel M. almost 4 yearsThanks so much for this! I've been spinning my wheels for hours trying to get this sorted; css-loader was giving me all sorts of problems and nothing on the internet helped. Thanks!!
-
Bjarne Gerhardt-Pedersen almost 4 yearsThanks!!! Finally an answer that actually worked exactly as required. I spent days on this problem!
-
Siva-Dev-Wizard almost 3 yearswow! thanks a lot mate. I was like searching this for weeks. I was like how webpack doesn't provide this. Even the docs say only one output can be given. Well finally! or i was about to add gulp in addition
-
Joe Coyle almost 2 yearsThank you so much, worked exactly as desired! I think a lot of dev hours would be saved if this solution was included in the official documentation for sass-loader @ npmjs.com/package/sass-loader