What does while (i --> 0) mean?
Solution 1
It should be read as
i-- > 0
So, what really happens is,
value of
i
will be checked if it greater than 0, if it is true then control will enter thewhile
block, if it is falsewhile
block will be skipped.Either way, the value of
i
will be decremented, immediately after the condition is checked.
Its always better to use for
loop, when we run a loop with a counter, like this
for (var i = els.length - 1; i >= 0; i -= 1) {
...
}
Please read more about whether ++
, --
is okay or not.
Solution 2
It's just weird spacing, should be
while((i--) > 0)
it's just post-decrementing and checking the condition. There was this humorous answer at the C++ question, but I think it got deleted
while (x --\
\
\
\
> 0) //i goes down to zero!
Or something like that, anyway
So if you had something like
var i=3;
while(i-->0){
console.log(i);
}
it would return
2
1
0
Solution 3
The code should actually be:
while (i-- > 0) {
where the loop will run if the value after the variable i
has been decremented is greater than zero.
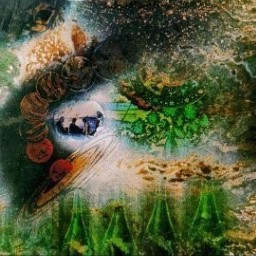
rvighne
Updated on July 23, 2022Comments
-
rvighne 2 months
I apologize if this a stupid question, but I cannot find the answer anywhere.
How does the following code work? (I realize that it loops over the elements of
els
)var i = els.length; while (i --> 0) { var el = els[i]; // ...do stuff... }
I have no idea what
-->
means. There is no documentation for it. Can someone enlighten me?