What is the easiest way to get an int in a console app?
Solution 1
#include <stdio.h>
int n;
scanf ("%d",&n);
See http://www.cplusplus.com/reference/clibrary/cstdio/scanf/
Solution 2
scanf()
is the answer, but you should certainly check the return value since many, many things can go wrong parsing numbers from external input...
int num, nitems;
nitems = scanf("%d", &num);
if (nitems == EOF) {
/* Handle EOF/Failure */
} else if (nitems == 0) {
/* Handle no match */
} else {
printf("Got %d\n", num);
}
Solution 3
Aside from (f)scanf
, which has been sufficiently discussed by the other answers, there is also atoi
and strtol
, for cases when you already have read input into a string but want to convert it into an int
or long
.
char *line;
scanf("%s", line);
int i = atoi(line); /* Array of chars TO Integer */
long l = strtol(line, NULL, 10); /* STRing (base 10) TO Long */
/* base can be between 2 and 36 inclusive */
strtol
is recommended because it allows you to determine whether a number was successfully read or not (as opposed to atoi
, which has no way to report any error, and will simply return 0 if it given garbage).
char *strs[] = {"not a number", "10 and stuff", "42"};
int i;
for (i = 0; i < sizeof(strs) / sizeof(*strs); i++) {
char *end;
long l = strtol(strs[i], &end, 10);
if (end == line)
printf("wasn't a number\n");
else if (end[0] != '\0')
printf("trailing characters after number %l: %s\n", l, end);
else
printf("happy, exact parse of %l\n", l);
}
Solution 4
The standard library function scanf is used for formatted input: %d int (the d is short for decimal)
#include <stdio.h>
int main(void)
{
int number;
printf("Enter a number from 1 to 1000: ");
scanf("%d",&number);
printf("Your number is %d\n",number);
return 0;
}
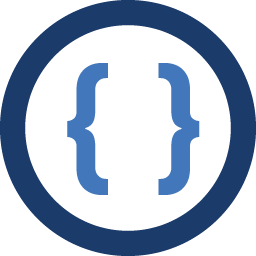
Admin
Updated on May 15, 2020Comments
-
Admin almost 4 years
I want to process user input as an integer, but it seems as though C has no way to get an int from stdin. Is there a function to do this? How would I go about getting an int from the user?
-
unwind almost 15 yearsThis looks very suspicious, it both stores the result of scanf() in i, and reads a value into i at the same time. Not recommendable.
-
P.Andrews over 8 yearsMine number goes to negative or random sometimes, why is this?This is if my integer is big, still within 32 characters but still big
-
PoVa almost 7 yearsAn integer is usually 2 bytes long. The leftmost bit is used to store the sign of the number (0 for positive, 1 for negative), so we're left with 15 bits for storing the number itself. Because of this, integers in C can range from -32768 (-2^15 or 1111111111111111) to 32768 (2^15 or 0111111111111111). When you enter a number larger than the largest possible value, the leftmost bit gets assigned a value and the integer no longer represents a value you were hoping for. If you want to enter bigger integers use
long int
or even 'long long int' as it can hold much bigger numbers.