What might be causing this buffer overflow?
Solution 1
This:
char a[4] = {'1', '2', '3'},b[4] = {'3', '2', '1'};
Creates two character arrays that hold the strings "123" and "321" respectively, but are not NULL-terminated. C-strings, by convention, must be NULL-terminated, or else functions that manipulate them (such as strcpy
) do not know where they end. You need to do this to give them the NULL terminator:
char a[4] = {'1', '2', '3', 0}, b[4] = {'3', '2', '1', 0};
^--------------------------^----> NULL terminators
(0 or '\0', NOT '0')
Also notice that the sizes of the arrays are changed from 3 to 4 to accommodate the ending NULL. You could also leave the size out to let the compiler determine what size to make the array by looking at how many things you put in the initialiser list:
char a[] = {'1', '2', '3', 0}, b[] = {'3', '2', '1', 0}; // sizes are left out
When you type in a string literal like this:
char a[] = "hello";
The compiler adds the NULL for you at the end, so what you're really doing is:
char a[] = "hello\0";
Which is why you don't have to do it when you type character arrays in double quotes.
Solution 2
The problem is that strcpy assumes it is looking at strings. The C convention for strings is that they are terminated by null characters. Your arrays are not, so strcpy doesn't know when to stop.
To fix this, either add a 4th null character or use strncpy.
The lack of null termination will also cause an issue when you printf.
Solution 3
both strcpy
and printf
expect c-style 0
-terminated strings. Your char
arrays are not 0
-terminated.
Therefore both functions will go havoc in the following blocks of memory until they find a '\0' byte.
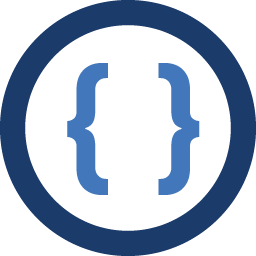
Admin
Updated on June 28, 2022Comments
-
Admin about 2 years
Hello I am trying to compile the following code,
#include <stdio.h> #include <string.h> int main() { int i; char a[3] = {'1', '2', '3'},b[3] = {'3', '2', '1'}; strcpy(a,b); for(i=0; i<3; i++) printf("%s",a); return 0; }
But I am getting the following error(
Buffer Overflow
)*** buffer overflow detected ***: ./prog terminated ======= Backtrace: ========= /lib/libc.so.6(__fortify_fail+0x48)[0xb7653ae8] /lib/libc.so.6[0xb7651b30] /lib/libc.so.6(__strcpy_chk+0x44)[0xb7650e14] ./prog[0x80484c9] ./prog[0x80483c1] ======= Memory map: ========
Now I don't get what is causing a buffer overflow here. Can anyone please help me out with it? Thanks in adcance.
-
Admin over 12 yearsOh, I just overlooked it. Thanks alot.