Why can't I put a delegate in an interface?
Solution 1
You can use any of these:
public delegate double CustomerDelegate(int test);
public interface ITest
{
EventHandler<EventArgs> MyHandler{get;set;}
CustomerDelegate HandlerWithCustomDelegate { get; set; }
event EventHandler<EventArgs> MyEvent;
}
Solution 2
A Delegate is just another type, so you don't gain anything by putting it inside the interface.
You shouldn't need to create your own delegates. Most of the time you should just use EventHandler, Func, Predicate, or Action.
May I ask what your delegate looks like?
Solution 3
this is a delegate TYPE decalaration...
public delegate returntype MyDelegateType (params)
this cant be declared in an interface as it is a type declaration
however using the type declaration above you CAN use a delegate instance
MyDelegateType MyDelegateInstance ( get; set;)
so delegate instances are OK but delegate type declarations aren't (in an interface)
Solution 4
A Delegate is a type which can't be declared in an interface. You might want to either use an event(if appropriate) or declare a delegate outside the interface but in the same namespace.
This link may help- When to Use Delegates Instead of Interfaces
Solution 5
The documentation clearly says that you can define a delegate in an interface:
An interface contains only the signatures of methods, delegates or events.
MSDN: interface (C# Reference)
However, in the remarks on the same page it says that an interface can contain signatures of methods, properties, indexers and events.
If you try to put a delegate in an interface, the compiler says that "interfaces cannot declare types."
The Ecma-334 standard (8.9 Interfaces) agrees with the remarks on that page and the compiler.
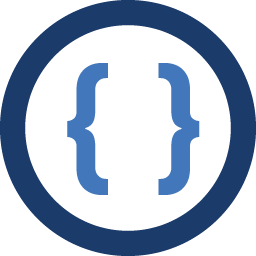
Admin
Updated on November 25, 2020Comments
-
Admin over 3 years
Why can't I add a delegate to my interface?
-
configurator about 15 yearsThat looks like an error in MSDN. That should be "the signatures of methods, properties or events", shouldn't it?
-
Jonathan Allen about 15 yearsWhy create CustomerDelegate when you can just use Func<int, double>?
-
eglasius about 15 yearsI know, I was just illustrating that you can use pretty much anything :)
-
Glenn Slayden almost 12 yearsHowever, note that you can name the arguments at the call site of a lambda function (but only if there are more than two):Action<int, int> f = (int i, int j) => { }; /* same as, */ var g = (Action<int, int>)((i, j) => { }); Oddly, you can't use 'var' when using the former syntax (which is when it is least needed).
-
Matthijs Wessels over 11 yearsThis answer actually also solved my problem that I could never find a nice way to use delegates in the situations where I wanted to use them. I.e. don't use 'm :).
-
diachedelic over 11 yearsIt gets difficult to understand parameters like Func<int, string[], bool>. Much more meaningful to have a name for that signature.
-
Kyle over 10 yearsWhen working across COM you actually have to use your own delegate as you cannot marshal generic types like Func<>, EventHandler<>, Action<>.
-
DavidGuaita over 7 yearsBest answer so far. To the point. I much I love when people goes to the point instead of writing speeches.