Why does the compiler still warn me about unsafe strtok even after I define _CRT_SECURE_NO_WARNINGS?
Solution 1
Your #define doesn't work because of the content of your precompiled header file (stdafx.h). The boilerplate one looks like this:
#pragma once
#include "targetver.h"
#include <stdio.h>
#include <tchar.h>
It is the last two #includes that cause the problem, those .h files themselves already #include string.h. Your #define is therefore too late.
Beyond defining the macro in the compiler settings instead, the simple workaround is to just move the #define into your stdafx.h file. Fix:
#pragma once
#define _CRT_SECURE_NO_WARNINGS
#include "targetver.h"
#include <stdio.h>
#include <tchar.h>
Solution 2
This may be an old post but I had a similar problem recently. I went into the project options -> C/C++ -> Preprocessor -> added _CRT_SECURE_NO_WARNINGS to the list of Preprocessor Definitions. This way you don´t have to put it in every file.
Solution 3
Try following.
#pragma warning (disable : 4996)
Note the error number is C4996.
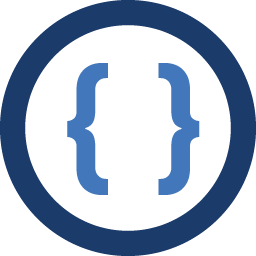
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
I am using Visual Studio Express 2012 for Windows Desktop.
I always get error
Error C4996: 'strtok': This function or variable may be unsafe. Consider using strtok_s instead. To disable deprecation, use _CRT_SECURE_NO_WARNINGS. See online help for details.
When I try to build the following:
#include "stdafx.h" #define _CRT_SECURE_NO_WARNINGS #include <iostream> #include <cstring> using namespace std; int main() { char the_string[81], *p; cout << "Input a string to parse: "; cin.getline(the_string, 81); p = strtok(the_string, ","); while (p != NULL) { cout << p << endl; p = strtok(NULL, ","); } system("PAUSE"); return 0; }
Why am I getting this error even though I define
_CRT_SECURE_NO_WARNINGS
, and how do I fix it?