Why don't I have to import a class I just made to use it in my main class? (Java)
Solution 1
It is because both are in same package(folder). They are automatically imported no need to write import statement for that.
Solution 2
You don't have to import classes that are in the same package as the current class.
Also, note that GradeBook.java is the name of the file. The (simple) name of the class is GradeBook. Every class should be in a package. If it is in the package com.foo.bar, the class name is com.foo.bar.GradeBook, and this is the name you must use when importing this class.
Read http://download.oracle.com/javase/tutorial/java/package/packages.html to learn more about packages.
Solution 3
It's all about packages. You are trying to use a class from the default package which does not need explicit import of the java file, ie GradeBook inside GradeBookTest
Here is where you can start with learning about packages :
and :
Solution 4
The classes located in the same package do not have to be imported, as they are visible to each other. You simply import
a class that is in another package:
import java.util.ArrayList;
Note that you are not importing the file, but the class.
Solution 5
Java doesn't use includes the way C does. Instead java uses a concept called the classpath, a list of resources containing java classes. The JVM can access any class on the classpath by name so if you can extend classes and refer to types simply by declaring them.
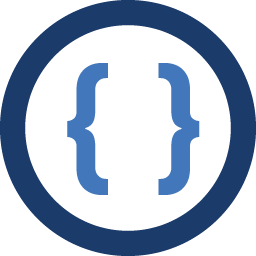
Admin
Updated on July 12, 2021Comments
-
Admin almost 3 years
I am currently learning Java using the Deitel's book Java How to Program 8th edition (early objects version).
I am on the chapter on creating classes and methods.
However, I got really confused by the example provided there because it consists of two separate .java files and when one of them uses a method from the other one, it did not import the class. It just created an object of that class from the other .java file without importing it first.
How does that work? Why don't I need to import it?
Here is the code from the book (I removed most comments, to save typing space/time...): .java class:
//GradeBook.java public class GradeBook { public void displayMessage() { System.out.printf( "Welcome to the grade book!" ); } }
The main .java file:
//GradeBookTest.java public class GradeBookTest { public static void main( String[] args) { GradeBook myGradeBook = new GradeBook(); myGradeBook.displayMessage(); } }
I thought I had to write
import GradeBook.java;
or something like that. How does the compiler know where GradeBook class and its methods are found and how does it know if it exists at all if we dont import that class?
I did lots of Googling but found no answer. I am new to programming so please tolerate my newbie question.
Thank you in advance.