Why variable can not start with a Number?
Solution 1
This is how PHP was designed. You can't start a variable name with a number.
What you can do is use underscore:
$_6 = $_REQUEST['6'];
EDIT: since variables start with $ in PHP, is would be possible to have variables starting with numbers, but that would be a bit confusing to most people since there is no other language that allows variables starting with numbers (or at least I don't know any).
But let's imagine variables starting with numbers are allowed.
Can you imagine a coworker saying to you: 23 equals 74? That is a bit confusing. Hearing n23 equals 74 makes more sense. You know n23 is a variable without having to explicitly say that.
Solution 2
Yes you can declare variables in PHP that start with a number:
${'1'} = 'hello';
This declares the variable with the variable name "1"
in PHP.
To access it, you need to use the brace syntax again:
echo ${'1'};
This is necessary because in PHP code verbatim you can not write:
echo $1;
That would give a syntax error.
Also can I create and use fields in Mysql with the same name?
Yes you can, it works similarly as in PHP. In Mysql as well you need to quote the number-only identifier for the column, the quoting differs:
mysql> create table test (`0` INT);
mysql> describe test;
+-------+---------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+---------+------+-----+---------+-------+
| 0 | int(11) | YES | | NULL | |
+-------+---------+------+-----+---------+-------+
Here the backticks are used (default Mysql configuration). Both the PHP manual and the Mysql manual explain the details of this, also there are many related questions to quoting identifiers both for PHP and Mysql on the Stackoverflow website.
Solution 3
It does not make sense. But you can still use the dynamic names of variables.
<?php
$name = 1;
$ $name = 'name';
// $ 1 = 'name'; // the same, but syntax not allowed
var_dump($ $name); // value of $1
var_dump(${$name});
var_dump($$name);
Solution 4
Well think about this:
$2d = 42;
$a = 2d;
What is a? 2.0? or 42?
Hint, if you don't get it, d after a number means the number before it is a double literal
There being difficulty in unambiguosly determining whether a numeric character in the compilation unit represented a literal or an identifier.
Languages where space is insignificant (like ALGOL and the original FORTRAN if I remember correctly) could not accept numbers to begin identifiers for that reason.
This goes way back - before special notations to denote storage or numeric base.
For a more detailed discussion visit Why can't variable names start with numbers?
Solution 5
To augment @hakre's answer you could also lose the quotes for brevity
${1}
but alas, you can not combine that with a string value. I did some experiments and apparently PHP expects either a string or a number value. And you can do anything within the curly brackets that eventually returns a number or a string value or is implicitly cast to either.
<?php
function eight (){
return 8;
}
${7+'fr'} = ${7*'fr'+1} = ${'hi'.((string)8).'hi'} = ${7.8910} = ${eight()} = ${(eight()==true)+4} = 'hi';
var_dump(get_defined_vars());
Outputs
array(8) { ["_GET"]=> array(0) { }
["_POST"]=> array(0) { }
["_COOKIE"]=> array(0) { }
["_FILES"]=> array(0) { }
["7.891"]=> string(2) "hi"
["7"]=> string(2) "hi"
["1"]=> string(2) "hi"
["hi8hi"]=> string(2) "hi"
["8"]=> string(2) "hi"
["5"]=> string(2) "hi" }
Addtionally I found something interesting:
<?php
${false} = 'hi';
${true} = 'bye';
var_dump(get_defined_vars());
Outputs
array(6) { ["_GET"]=> array(0) { }
["_POST"]=> array(0) { }
["_COOKIE"]=> array(0) { }
[""]=> string(2) "hi"
["1"]=> string(3) "bye" }
Which means false
evaluates to an empty string and not 0 and true
to 1.
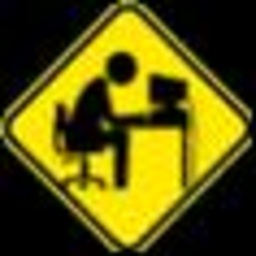
Comments
-
Methew about 1 year
I am working in PHP, I have to define variables in sequence to save in Mysql. Both field name and variable name must be same in my case.
Can I declare a variable like this
$1 OR $2 etc
If not why not and if yes why yes?
I tried:
$id = 0; $6 = $_REQUEST['6']; $7 = $_REQUEST['7']; $8 = $_REQUEST['8']; $xary = array('6','7','8','9') $oAppl->save_record("tablename", $id, "id");
which give me error.
Also can I create and use fields in Mysql with the same name?
-
hakre over 9 yearsPHP does allow variable names that consist of numbers only. Same identifiers in Mysql - It's just the question if that is useful. But to actually answer the concrete question: stackoverflow.com/a/19054047/367456
-
TylerH over 9 yearsLuckily programming languages are not spoken languages, @RobotMess :-P That way we have the benefit of knowing whether the co-worker is saying
23 == 74
or saying23 = 74
. Of course I still get your point with that example. :-) -
Naughty.Coder almost 8 yearsThis is awfully wrong in this context. This was an answer to C syntax .. PHP variables start with $ , so your example is actually syntactically wrong. ($2d not 2d)
-
Dreaded semicolon over 7 yearsPHP variables start with $. there is no reason not to allow numbers. except like the bride that chops the end of the roast.
-
Tama about 6 yearsDecoding a json that have a variable name starting with a digit, the only way to access it is to use the syntax proposed by hakre. Having $json = '{"52WeekChange":"0,3"}'; You can access $decoded->{'52WeekChange'};
-
Ogier Schelvis over 5 yearsI thought of this aswell. This is the closest you can get to it. But technically your variable starts with a curly brace ;-)