WPF MVVM ListBox.ItemTemplate CheckBox IsChecked binding
I'm guessing your Item source of AllPermissions is a collection of Permission objects. So just make sure that in addition to the DisplayName that it also has something on there that determines whether the role has the permission:
public class Permission : ViewModelBase
{
private string displayName;
private bool roleHasPermission;
public string DisplayName
{
get
{
return this.displayName;
}
set
{
this.displayName = value;
this.RaisePropertyChanged(() => this.DisplayName);
}
}
public bool RoleHasPermission
{
get
{
return this.roleHasPermission;
}
set
{
this.roleHasPermission = value;
this.RaisePropertyChanged(() => this.RoleHasPermission);
}
}
}
so then Bind IsChecked to RoleHasPermission.
Now I'm guessing at the moment that you are loading the available permissions from somewhere and they are currently ignorant of if the role has thepermission, so when you are loading the AllPermissions, calculate whether the rolehas the permission.
I have assumed you have inherited from a base class that has a RaisePropertyChanged event on it to notify the view when the value has been updated. (such as provided for you if you use mvvm light or other frameworks, or you can write your own) Also if you want to be able to edit the permission by checking/unchecking the check box, then remember to set the binding Mode=TwoWay ie:
<ListBox
...
ItemsSource="{Binding AllPermissions}">
<ListBox.ItemTemplate>
<DataTemplate>
<CheckBox Content="{Binding DisplayName}"
IsChecked="{Binding RoleHasPermission, Mode=TwoWay}"/>
</DataTemplate>
</ListBox.ItemTemplate>
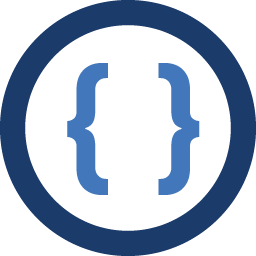
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
The scenario I'm working with is editing Roles and Permissions. In a list box I want to list all defined Permissions and check the Permissions the selected Role has been assigned. The Role selection occurs in a separate list.
I have a simple view that contains a list box that displays all permissions:
<ListBox ... ItemsSource="{Binding AllPermissions}"> <ListBox.ItemTemplate> <DataTemplate> <CheckBox Content="{Binding DisplayName}" IsChecked="???"/> </DataTemplate> </ListBox.ItemTemplate> </ListBox>
The ItemsSource is one set of Permissions and the selected role's Permissions is a different set. How would I bind the IsChecked value to the intersection of the sets (i.e., if the Permission in the ListBox is also in the selected role's Permissions then the box should be checked)?