Android beginner: Touch events in android gridview
Solution 1
Use the OnTouchListener
in this way. Read up on MotionEvent types like ACTION_UP
, ACTION_MOVE
, and ACTION_DOWN
which mean that the key was pressed here, mouse was moved, or key was unpressed here...
public void addListenerToGrid() {
gridView = (GridView) findViewById(R.id.gridView1);
gridView.setOnTouchListener(new OnTouchListener() {
public boolean onTouch(View v, MotionEvent me) {
int action = me.getActionMasked();
float currentXPosition = me.getX();
float currentYPosition = me.getY();
int position = gridView.pointToPosition((int) currentXPosition, (int) currentYPosition);
if (action == MotionEvent.ACTION_UP) {
// Key was pressed here
}
return true;
}
Solution 2
Following on from your code, as jaydeepfifadra suggested you can use the setOnItemClickListener method of the gride view rather than the image itself. (Not to say that that isn't possible but i've not tried it).
gridView.setOnItemClickListener(
new OnItemClickListener(){
public void onItemClick(AdapterView<?> arg0, View view, int arg2, long arg3) {
((Image)view.setSelected(!(Image)view.getSelected()));
}
});
);
Now i'm not 100% sure if the following will work, but in the above i've set the view.setSelected() state of the image to toggle. I'm sort of guessing that you can set the image resource to a drawable selector. i.e.
make your R.drawable.sample_2 resources selectors, such as:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_selected="true" android:drawable="@drawable/image_selected" />
<item android:drawable="@drawable/image_unselected" />
</selector>
However, whilst this allows you to detect the single tap that would toggle the selected state. It doesn't cover the double tap. There also doesn't appear to be a setOnDoubleClickItemListener() method.
As such it might be required for your specific situation that you implement a GestureDetector and implement your own SimpleOnGestureListener class (MyGestureListener extends SimpleOnGestureListener) that manages the double and single tap events your looking for.
You can see an example of implementing the gesture listener and detector here.
how to implement both ontouch and also onfling in a same listview?
You would set the gestureListener as the onTouchListener of your grid view.
Solution 3
Use the onItemSelectListener
of gridView.
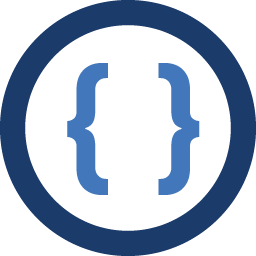
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am using the following code to do things with gridview(slightly modified from http://developer.android.com/resources/tutorials/views/hello-gridview.html). I want to replace the onClicklistener and the onClick() method with their "touch" equivalents i.e. touchlistener and onTouch() so that when i touch an element in the gridview the image of the element changes and a double touch on the same element takes it back to the orginal state.
How do I do this? I can't get my code to do this. The clicklistener works to some extent but the touch isn't. Please help.
public class ImageAdapter extends BaseAdapter { private Context mContext; public ImageAdapter(Context c) { mContext = c; } public int getCount() { return mThumbIds.length; } public Object getItem(int position) { return null; } public long getItemId(int position) { return 0; } // create a new ImageView for each item referenced by the Adapter public View getView(int position, View convertView, ViewGroup parent) { ImageView imageView; if (convertView == null) { // if it's not recycled, initialize some attributes imageView = new ImageView(mContext); imageView.setLayoutParams(new GridView.LayoutParams(85, 85)); imageView.setScaleType(ImageView.ScaleType.CENTER_CROP); imageView.setPadding(8, 8, 8, 8); imageView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { if(position==0) { //do this } else { //do this } } }); } else { imageView = (ImageView) convertView; } imageView.setImageResource(mThumbIds[position]); return imageView; } // references to our images private Integer[] mThumbIds = { R.drawable.sample_2, R.drawable.sample_3, R.drawable.sample_4, R.drawable.sample_5, R.drawable.sample_6, R.drawable.sample_7, R.drawable.sample_0, R.drawable.sample_1, R.drawable.sample_2, R.drawable.sample_3, R.drawable.sample_4, R.drawable.sample_5, R.drawable.sample_6, R.drawable.sample_7, R.drawable.sample_0, R.drawable.sample_1, R.drawable.sample_2, R.drawable.sample_3, R.drawable.sample_4, R.drawable.sample_5, R.drawable.sample_6, R.drawable.sample_7 }; }
-
Mr. Fish over 6 yearsSince onItemClickListener does not catch every single touch event, this is the only solution to handle very fast touches.