Angular2 include html from server into a div
Solution 1
Angular security Blocks dynamic rendering of HTML and other scripts. You need to bypass them using DOM Sanitizer.
Read more here : Angular Security
DO below changes in your code :
// in your component.ts file
//import this
import { DomSanitizer } from '@angular/platform-browser';
// in constructor create object
constructor(
...
private sanitizer: DomSanitizer
...
){
}
someMethod(){
const headers = new HttpHeaders({
'Content-Type': 'text/plain',
});
const request = this.http.get<string>('https://developer.mozilla.org/en-US/docs/Learn/HTML/Forms/Your_first_HTML_form', {
headers: headers,
responseType: 'text'
}).subscribe(res => this.htmlString = res);
this.htmlData = this.sanitizer.bypassSecurityTrustHtml(this.htmlString); // this line bypasses angular security
}
and in HTML file ;
<!-- In Your html file-->
<div [innerHtml]="htmlData">
</div>
Here is the working example of your requirement :
Solution 2
This should do it:
First in your component.ts get the html with a http request:
import { Component, OnInit } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { map } from 'rxjs/operators'
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
constructor(private http: HttpClient) { }
htmlString: string;
ngOnInit() {
const headers = new HttpHeaders({
'Content-Type': 'text/plain',
});
const request = this.http.get<string>('https://developer.mozilla.org/en-US/docs/Learn/HTML/Forms/Your_first_HTML_form', {
headers: headers,
responseType: 'text'
}).subscribe(res => this.htmlString = res);
}
}
And in your component.html simply use a one way data binding:
<div [innerHTML]="htmlString"></div>
Related videos on Youtube
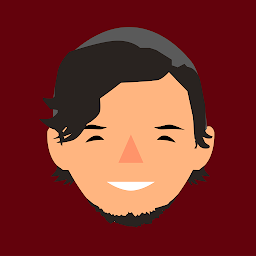
Sergio Mendez
Updated on June 04, 2022Comments
-
Sergio Mendez almost 2 years
I got a serie of html in my server. For example:
And I trying to include those files into a
<div>
in my angular2 v4 app. For example:component.ts
public changePage(name: string) { switch (name) { case 'intro': this.myHtmlTemplate = 'http://docs.example.com/intro.html'; break; case 'page1': this.myHtmlTemplate = 'http://docs.example.com/page1.html'; break; case 'page2': this.myHtmlTemplate = 'http://docs.example.com/page2.html'; break; } }
component.html
<div [innerHtml]="myHtmlTemplate"></div>
but it doesnt work. I tried the following solutions:
Angular4 Load external html page in a div
Dynamically load HTML template in angular2
but it doesn't work for me. Can somebody help me with this problem please ?
-
msanford almost 5 yearsThis is not at how you should be doing this. Look at concretepage.com/angular-2/…
-
Sergio Mendez almost 5 yearsthank you very much, I'm avoiding the <iframe> becouse I have some styles into the angular App, that I need to appear into the template.html from server. What I need is a way to go to docs.example.com/page2.html and practically convert that file in plain text to put using [innerHtml] so the angular styles aply to this section
-
Sergio Mendez almost 5 yearsSure I tried. Then the "responseType: 'text'" returns the following error: Type '"text"' is not assignable to type '"json"'.ts(2322) client.d.ts(639, 9): The expected type comes from property 'responseType' which is declared here on type ...
-
Sergio Mendez almost 5 yearsI like your solution. Unfortunately it doesn't work for all .html files. Even in the stackblitz demo, returns console errors. If you have another solution in any other way (except an iframe), I would like to know. Thank you
-
programoholic almost 5 years@SergioMendez that is because the website didn't send the html response (may be due to cross origin request). If you have list of the websites which returns the html content. The above solution will definitely work.
-
Sergio Mendez almost 5 yearsThank you very much. You Really helpme to find the solution.
-
Marco over 3 yearsyou have to set "responseType: 'text' as 'json'" to fool the typescript transpiler