Application of TryParse in C#
Solution 1
The code is as follows to determine whether the string val
is a valid Boolean value and use it to set the Checked
property if so. You need to decide what action you would take if it does not represent a valid value.
bool result;
if (bool.TryParse(val, out result))
{
// val does represent a Boolean
checkBox.Checked = result;
}
else
{
// val does not represent a Boolean
}
Solution 2
Assuming that if its not a valid boolean, you don't want it checked:
bool result = false;
bool.TryParse(val, out result);
checkBox.Checked = result;
Solution 3
bool z = false;
if(Boolean.TryParse(val, out z))
{
checkBox.Checked = z;
}
Just a note: Parse and convert are different operations and may lead to different results.
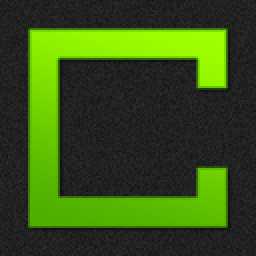
Cᴏʀʏ
I work at Corporate Technology Solutions, Inc., a software consulting firm that specializes in custom software development for the financial services industries in the Greater Milwaukee Area in Wisconsin. I earned a Bachelor of Science degree in Software Engineering from Milwaukee School of Engineering. As a consultant and engineer, typical projects for me revolve around full-stack web application development. My strengths include: gathering functional and technical requirements, database design and administration (ssis, sql-server), development (c#, vb.net, aspnet-mvc, sql, javascript, jquery and more), testing, integration, and training. In my spare time I like to read, relax, sample great Wisconsin beer, and of course, contribute to Stack Overflow! Some achievements I'm proud of (Stack Overflow): Usually in the top 1,000 users for all time rank by reputation 38/40 candidate score (if I were to ever run for moderator) c# – gold badge jquery – gold badge javascript – silver badge sql – silver badge sql-server – silver badge I never intend to give bad advice. If you find that any of my answers are counter-productive or just plain wrong, please leave a comment! I'm more than happy to fix or delete answers if they aren't up to snuff.
Updated on November 18, 2022Comments
-
Cᴏʀʏ about 2 hours
When 'val' below is not a
bool
I get an exception, I believe I can useTryParse
but I'm not sure how best to use it with my code below. Can anyone help?checkBox.Checked = Convert.ToBoolean(val);
Thanks
-
Servy over 9 years1) This doesn't answer the question that is asked here. 2) If you find yourself posting the same answer to lots of different questions you're doing something wrong. Either the questions really are different and the answer doesn't apply to all of them (which is the case here), or the questions are duplicates and should just have all but one closed as a duplicate of the best one.
-
Mick Bruno over 9 yearsI think that this is a valid reply to the question. They asked why TryParse didn't work, I gave a routine that would do what they wanted. Perhaps I should have added the usage: bool shouldCheck; TryParseBool(val, out shouldCheck); checkBox.Checked = shouldCheck;