Assembly Basics: Output register value
28,879
Solution 1
mov ah, 00h ; display function here?
No, the single-char display function is at AH=2 / int 21h
Since your BL register contains only a small value (9) all it would have taken was:
mov ah, 02h
mov dl, bl
add dl, "0" ; Integer to single-digit ASCII character
int 21h
If values become a bit bigger but not exceeding 99 you can get by with:
mov al, bl ; [0,99]
aam ; divide by 10: quotient in ah, remainder in al (opposite of DIV)
add ax, "00"
xchg al, ah
mov dx, ax
mov ah, 02h
int 21h
mov dl, dh
int 21h
A solution that doesn't use the AAM
instruction:
mov al, bl ; [0,99]
cbw ; Same result as 'mov ah, 0' in this case
mov dl, 10
div dl ; Divides AX by 10: quotient in al, remainder in ah
add ax, "00"
mov dx, ax
mov ah, 02h
int 21h
mov dl, dh
int 21h
Solution 2
In emu8086 you can use a ready-made macro and procedure for that purpose.
Example:
include 'emu8086.inc' ; Include useful macros and procedures
.model small
.stack
.data
var1 db 6
var2 db 2
var3 db 7
.code
DEFINE_PRINT_NUM ; Create procedure PRINT_NUM
DEFINE_PRINT_NUM_UNS ; Create procedure PRINT_NUM_UNS
crlf proc
mov ah, 2
mov dl, 13
int 21h
mov dl, 10
int 21h
ret
crlf endp
main proc
mov ax, @data
mov ds, ax
; test output: 54321 & -11215
mov ax, 54321
call PRINT_NUM_UNS ; Print AX as unsigned number
call crlf
mov ax, 54321
call PRINT_NUM ; Print AX as signed number
call crlf
mov bl, var1
add bl, var2
add bl, var3
mov ax, bx ; AX contains the number for PRINT_NUM
xor ah, ah ; Could contain crap
call PRINT_NUM
call crlf
mov ax, 4c00h
int 21h
main endp
end main
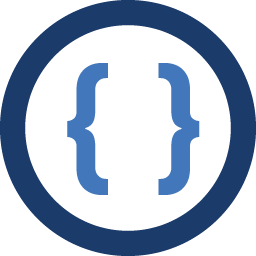
Author by
Admin
Updated on April 20, 2020Comments
-
Admin about 4 years
I just started learning assembly language and I am already stuck on the part to "display the decimal values stored in a register on the screen". Im using the emu8086, any help would be appreciated! :)
.model small ;Specifies the memory model used for program to identify the size of code and data segments org 100h ;allocate 100H memory locations for stack .data ;the segment of the memory to declare/initialze the variables var1 db 0006 var2 db 0002 var3 db 0001 .code ;start of the code segment main proc ;start of the first procedure mov bl, var1 add bl, var2 add bl, var3 mov ah, 00h ; display function here? mov dl, bl ; output the bl register's value? int 21h mov ah, 4ch ;exit DOS function int 21h endp ;end of the first procedure end main ;end of the complete assembly program ret