C#: How do you save a list of items like a Combobox to the .NET Settings file?
Solution 1
The only collection type of use that the Settings Designer lets you use is System.Collections.ArrayList. If you do use an ArrayList, all of its elements' types must be serializable (have the [Serializable] attribute or implement System.Runtime.Serialization.ISerializable.)
Here's some code to get data from an ArrayList (named cboCollection) in Settings into a combo box and back.
private void Form1_Load(object sender, EventArgs e)
{
if (Settings.Default.cboCollection != null)
this.comboBox1.Items.AddRange(Settings.Default.cboCollection.ToArray());
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
ArrayList arraylist = new ArrayList(this.comboBox1.Items);
Settings.Default.cboCollection = arraylist;
Settings.Default.Save();
}
//A button to add items to the ComboBox
private int i;
private void button1_Click(object sender, EventArgs e)
{
this.comboBox1.Items.Add(i++);
}
Solution 2
If you are talking about the Application User Settings, I'd loop through the combobox and save the values in a delimited string:
StringBuilder sb = new StringBuilder();
foreach(var item in combo.Items){
sb.Append(item.ToString() + ";");
}
Properties.Settings.MyListSetting = sb.ToString();
Please excuse if the above code isn't perfect, It's just an example.
Hope that helps!
Solution 3
The Windows Forms objects are not serializable. Therefore you cannot serialize and store them in a file with a binaryformatter. You need to store the combobox values manually in a file.
string comboboxFileName = @"c:\workDir\settings.settings";
private void saveComboboxInFile (String comboboxFileName )
{
//--------------------------------------------------------
//- Store the combobox values in a file. 1 value = 1 line
//--------------------------------------------------------
try
{
using (StreamWriter comboboxsw = new StreamWriter(comboboxFileName))
{
foreach (var cfgitem in comboBox.Items)
{
comboboxsw.WriteLine(cfgitem);
}
} // End Using`
}
catch (Exception e)
{
//process exception
}
}
private void reloadCombboxFromFile (string comboboxFileName )
{
//-------------------------------------------------
//- Read the values back into the combobox
//-------------------------------------------------
try
{
using (StreamReader comboboxsr = new StreamReader(comboboxFileName))
{
while (!comboboxsr.EndOfStream)
{
string itemread = comboboxsr.ReadLine();
comboBox.Items.Add(itemread);
}
} // End Using
}
catch (DirectoryNotFoundException dnf)
{
// Exception Processing
}
catch (FileNotFoundException fnf)
{
// Exception Processing
}
catch (Exception e)
{
// Exception Processing
}
}
Related videos on Youtube
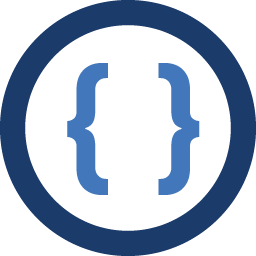
Admin
Updated on April 16, 2022Comments
-
Admin about 2 years
C#: How do you save a list of items like a Combobox to the .NET Settings file?