C# - How to extract the file name and extension from a path?
67,680
Solution 1
System.IO
has different classes to work with files and directories. Between them, one of the most useful one is Path
which has lots of static helper methods for working with files and folders:
Path.GetExtension(yourPath); // returns .exe
Path.GetFileNameWithoutExtension(yourPath); // returns File
Path.GetFileName(yourPath); // returns File.exe
Path.GetDirectoryName(yourPath); // returns C:\Program Files\Program
Solution 2
You're looking for Path.GetFileName(string)
.
Solution 3
With the last character search you can get the right result.
string path = "C:\\Program Files\\Program\\fatih.gurdal.docx";
string fileName = path.Substring(path.LastIndexOf(((char)92))+ 1);
int index = fileName.LastIndexOf('.');
string onyName= fileName.Substring(0, index);
string fileExtension = fileName.Substring(index + 1);
Console.WriteLine("Full File Name: "+fileName);
Console.WriteLine("Full File Ony Name: "+onyName);
Console.WriteLine("Full File Extension: "+fileExtension);
Output:
Full File Name: fatih.gurdal.docx
Full File Ony Name: fatih.gurdal
Full File Extension: docx
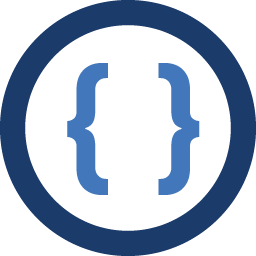
Author by
Admin
Updated on January 04, 2021Comments
-
Admin over 3 years
So, say I have
string path = "C:\\Program Files\\Program\\File.exe";
How do I get only "File.exe"? I was thinking something with split (see below), but what I tried doesn't work...
This is my code.
List<string> procs = new List<string>(); //Used to check if designated process is already running foreach (Process prcs in Process.GetProcesses()) procs.Add(prcs.ProcessName); //Add each process to the list foreach (string l in File.ReadAllLines("MultiStart.txt")) //Get list of processes (full path) if (!l.StartsWith("//")) //Check if it's commented out if (!procs.Contains(l.Split('\\')[l.Split('\\').Length - 1])) //Check if process is already running Process.Start(l);
I'm probably just being a noob. ._.